A Perfect Match: Python And JSON
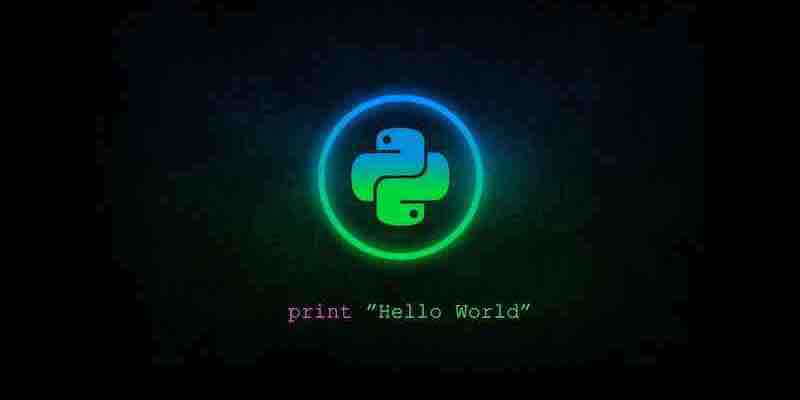
In Python, we work with JSON data using the json
module that is a part of the standard library. Here are some examples demonstrating how to use the json
module to encode Python data to JSON format and decode JSON data to Python data:
1. Importing the JSON Module
First, you need to import the json
module:
import json
2. Converting Python Data to JSON (Serialization)
You can convert Python data structures to JSON format using the json.dumps()
method (returns a JSON string) or the json.dump()
method (writes JSON data to a file).
2.1 Using json.dumps()
import json
# Python dictionary
data = {
"name": "John",
"age": 30,
"city": "New York"
}
# Convert Python dictionary to JSON string
json_data = json.dumps(data)
print(json_data)
2.2 Using json.dump()
import json
data = {
"name": "John",
"age": 30,
"city": "New York"
}
# Write JSON data to a file
with open('data.json', 'w') as json_file:
json.dump(data, json_file)
3. Converting JSON Data to Python (Deserialization)
You can convert JSON data to Python data structures using the json.loads()
method (parses a JSON string) or the json.load()
method (reads JSON data from a file).
3.1 Using json.loads()
import json
# JSON string
json_data = '{"name": "John", "age": 30, "city": "New York"}'
# Parse JSON string to Python dictionary
data = json.loads(json_data)
print(data)
3.2 Using json.load()
import json
# Read JSON data from a file
with open('data.json', 'r') as json_file:
data = json.load(json_file)
print(data)
4. Formatting JSON Output
You can format the JSON output using the indent
parameter in the json.dumps()
and json.dump()
methods:
import json
data = {
"name": "John",
"age": 30,
"city": "New York"
}
# Convert Python dictionary to formatted JSON string
json_data = json.dumps(data, indent=4)
print(json_data)
This will give you a nicely formatted JSON string with an indentation of 4 spaces.
Remember to handle exceptions using try-except blocks to catch any errors that might occur during the JSON encoding and decoding processes.