Exploring Python Variables
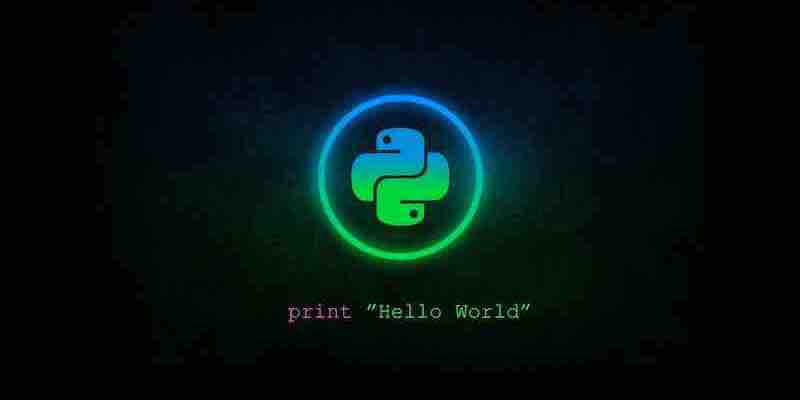
In the vast landscape of programming, understanding variables is a fundamental step. Variables are the cornerstone of Python, just as in any other programming language.
In Python, a variable stores information that can be referenced and manipulated throughout a program. Unlike many other languages, Python does not require the explicit declaration of the data type of a variable; it is dynamically inferred. This feature makes Python both flexible and easy to work with. Let’s dive deeper into various aspects concerning Python variables.
1. Variable Assignment
Assigning a value to a variable in Python is straightforward. You use the equals sign (=) to give a value to a variable name.
x = 5
name = "Alice"
In the above code snippet, 5
is assigned to x
, and the string "Alice"
is assigned to name
.
2. Multiple Assignments
Python allows you to assign values to multiple variables in a single line, making your code more concise.
x, y, z = 5, 3.5, "Hello"
Here, 5
is assigned to x
, 3.5
to y
, and "Hello"
to z
.
3. Data Types
Though Python is dynamically typed, it’s essential to understand the different data types that can be assigned to variables. Here are examples of other data-type assignments:
integer_var = 10 # Integer data type
float_var = 20.5 # Float data type
string_var = "Python" # String data type
list_var = [1, 2, 3] # List data type
tuple_var = (1, 2, 3) # Tuple data type
dictionary_var = {"a": 1, "b": 2} # Dictionary data type
4. Variable Names
Variable names in Python can be any length and can consist of uppercase and lowercase letters (A-Z
, a-z
), digits (0-9
), and the underscore character (_
). However, they cannot start with a number.
my_variable = 5
variable2 = "Hello"
_this_is_a_variable = 10
5. Constants
In Python, constants are variables whose values are not meant to be changed throughout the execution of the program. By convention, constant variable names are written in uppercase letters.
PI = 3.14159
MAX_SIZE = 100
6. Global Variables
Global variables are defined outside of any function, and any role in the program can access them.
global_var = "I am a global variable"
def my_function():
print(global_var)
my_function() # Output: I am a global variable
7. Deleting Variables
You can remove a variable entirely using the del
statement, which frees up the memory space occupied by that variable.
x = 5
print(x) # Output: 5
del x
print(x) # This will cause an error because x no longer exists
Understanding variables is a stepping stone in the journey of learning Python programming. This article has explored various facets of Python variables, along with accompanying code samples. As you progress, you’ll find that a firm grasp of variables will pave the way for more complex and dynamic programming feats in Python. Happy coding!