Unleashing the Power of Python Generators: A Journey into Efficient Iterations
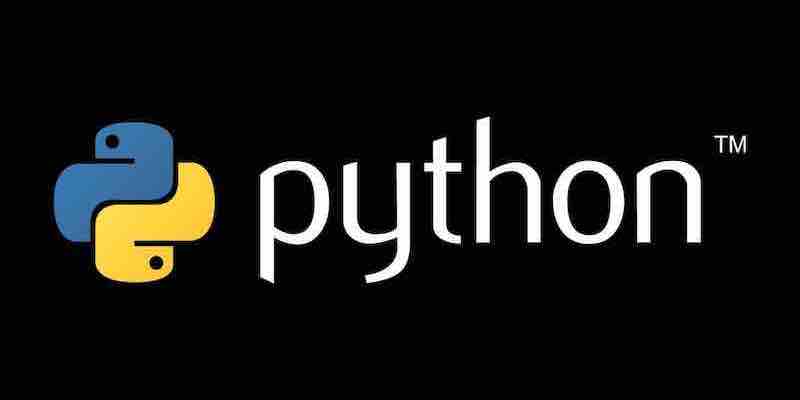
Python has carved a niche for itself in the dynamic and ever-evolving programming landscape, mainly owing to its readability, efficiency, and robust collection of libraries and tools. Among its diverse features, Python’s generators testify to the language’s commitment to clean, efficient, and powerful code. In this article, we explore the world of Python generators, illustrating their utility and efficiency with handy code examples.
Understanding Generators
Generators are iterable in Python, similar to lists or tuples. However, they don’t allow indexing but can still be iterated through with for loops. They are generated by functions that use the yield statement to return a sequence of values lazily, meaning they produce one weight at a time and can save memory when dealing with extensive data collection.
Here’s a simple example of a generator function that yields numbers from 0 to n-1:
def count_up_to(n):
count = 0
while count < n:
yield count
count += 1
# Using the generator
for number in count_up_to(5):
print(number)
Creating Generators
Creating a generator is as simple as defining a function and replacing the return statement with a yield
statement. The yield statement suspends the function’s execution and sends a value back to the caller but retains enough state to enable function resumption right where it left off.
Here is a primary generator that yields even numbers:
def even_numbers_up_to(n):
for number in range(n):
if number % 2 == 0:
yield number
# Using the generator
for number in even_numbers_up_to(10):
print(number)
Generators with Expressions
Besides generator functions, Python also supports generator expressions, which are even more concise and are created using the same syntax as list comprehensions but with parentheses instead of square brackets.
# Generator expression to create even numbers
even_numbers = (x for x in range(10) if x % 2 == 0)
# Using the generator
for number in even_numbers:
print(number)
Chaining Generators
Generators can be chained together to create powerful and efficient data processing pipelines. Here’s an example where two generators are chained to process data:
def first_generator(data):
for element in data:
if element % 2 == 0:
yield element
def second_generator(data):
for element in data:
yield element * element
# Chaining generators
data = range(10)
pipeline = second_generator(first_generator(data))
for value in pipeline:
print(value)
Benefits of Using Generators
- Memory Efficiency: Generators are memory-efficient as they only generate one item at a time rather than storing all the items in memory.
- Lazy Evaluation: Generators evaluate items lazily, which means they are considered one at a time and only when needed.
- Cleaner Code: Generators can lead to cleaner code by separating data processing code into independent, reusable generators.
Summary
Python generators offer an elegant and robust solution for creating iterable objects focusing on memory efficiency and clean code. By understanding and utilizing generator functions and expressions, developers can create data pipelines that are efficient but also concise and readable.
As you venture further into your Python journey, integrating generators into your coding practices can help you craft robust and efficient code, taking your Python prowess to the next level.