AWS EKS: The Easy Way to Run Kubernetes
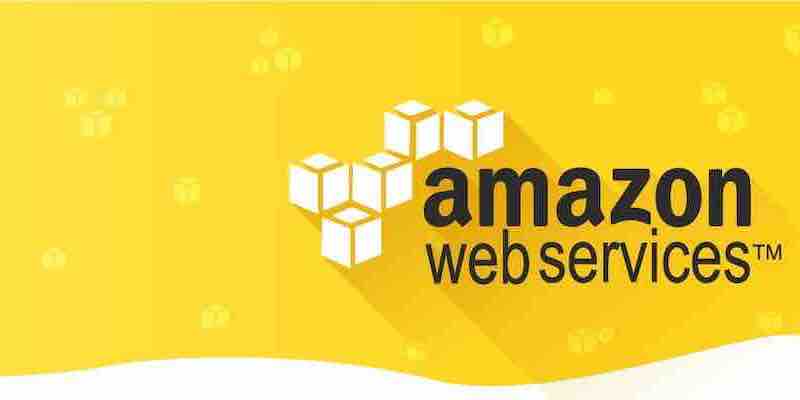
Subtitle: A managed Kubernetes service that makes it easy to run Kubernetes on AWS.
Introduction
Kubernetes is a powerful container orchestration platform that can help you deploy and manage your containerized applications. However, managing Kubernetes can be complex and time-consuming.
AWS EKS is a managed Kubernetes service that makes it easy to run Kubernetes on AWS. EKS takes care of the underlying infrastructure, so you can focus on building and deploying your applications.
What is AWS EKS?
AWS EKS is a managed Kubernetes service that makes it easy to run Kubernetes on AWS. EKS takes care of the underlying infrastructure, so you can focus on building and deploying your applications.
EKS provides several features that make it easy to run Kubernetes on AWS, including:
- Automatic cluster provisioning: EKS automatically provisions Kubernetes clusters for you.
- High availability: EKS clusters are highly available, so your applications will be up and running even if one of the nodes goes down.
- Security: EKS clusters are secure, so you can be confident that your applications are protected.
- Scalability: EKS clusters can be scaled up or down as needed, so you can easily accommodate changes in traffic.
How does AWS EKS work?
EKS works by providing a managed Kubernetes control plane. The control plane is responsible for managing the Kubernetes nodes in your cluster. EKS also provides several features that make interacting with the control plane easy, such as the AWS CLI and the AWS SDKs.
When you create an EKS cluster, EKS provides a set of nodes. The nodes are running the Kubernetes control plane and your application containers. EKS also manages the nodes for you, so you don’t have to worry about node provisioning, scaling, or maintenance.
Benefits of using AWS EKS
There are many benefits to using AWS EKS, including:
- Ease of use: EKS makes it easy to run Kubernetes on AWS. You don’t have to worry about managing the underlying infrastructure, so you can focus on building and deploying your applications.
- High availability: EKS clusters are highly available, so your applications will be up and running even if one of the nodes goes down.
- Security: EKS clusters are secure, so you can be confident that your applications are protected.
- Scalability: EKS clusters can be scaled up or down as needed, so you can easily accommodate changes in traffic.
How to use AWS EKS
To use AWS EKS, you first need to create an EKS cluster. You can do this using the AWS Management Console, the AWS CLI, or the AWS SDKs.
Once you have created a cluster, you can deploy your applications to the cluster. You can do this using the Kubernetes command-line tools or the AWS SDKs.
Python libraries for AWS EKS
There are several Python libraries that you can use to interact with AWS EKS. These libraries can help you to:
- Create and manage EKS clusters.
- Deploy applications to EKS clusters
- Manage EKS resources
Some of the most popular Python libraries for AWS EKS include:
boto3
: The official AWS SDK for Pythoneksctl
: A command-line tool for creating and managing EKS clusterskubernetes
: The official Python library for Kubernetes
Python code samples
Here is a simple Python code sample that shows how to create an EKS cluster:
import boto3
client = boto3.client('eks')
cluster = client.create_cluster(
Name='my-cluster',
Version='1.21',
NodeCount=3
)
print(cluster)
Here is a simple Python code sample that shows how to deploy an application to an EKS cluster:
“`python
import boto3
import kubernetes
client = boto3.client(‘eks’)
cluster = client.describe_cluster(Name=’my-cluster’)
kubeconfig = client.get_kubeconfig(ClusterName=’my-cluster’)
kubernetes.config.load_kube_config(kubeconfig)
app = kubernetes.client.V1Deployment(
metadata=kubernetes.client.V1ObjectMeta(name=’my-app