Beautiful Soup: A Python library for web scraping
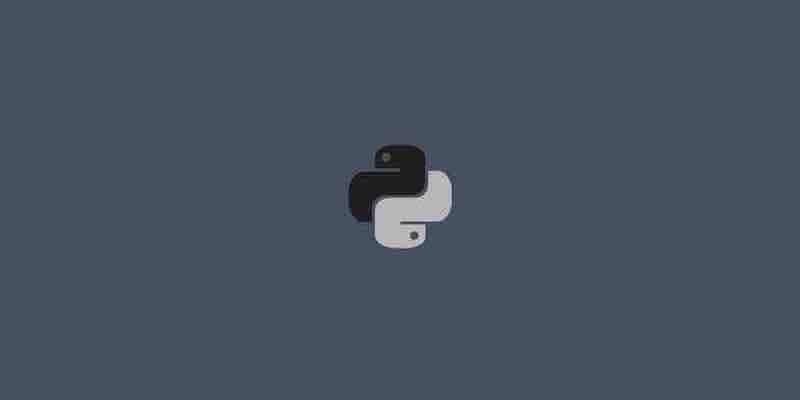
Beautiful Soup is a Python library for parsing HTML and XML documents. It provides a simple and easy-to-use API for extracting data from HTML, which is helpful for web scraping.
Beautiful Soup was first released in 2004 by Leonard Richardson. It was initially developed as a research project at the University of North Carolina at Chapel Hill. Beautiful Soup has since become a popular library for web scraping and is used by many researchers and developers.
Benefits/Drawbacks
Beautiful Soup has several benefits, including:
- It is easy to use. Beautiful Soup provides a simple API that makes it easy to extract data from HTML.
- It is versatile. Beautiful Soup can be used to extract data from various HTML documents.
- It is well-documented. Beautiful Soup is well-documented, making learning how to use it easy.
Beautiful Soup also has a few drawbacks, including:
- It can be slow. Beautiful Soup can be slow for large HTML documents.
- It is not as powerful as some other web scraping libraries.
- It is not as well-maintained as some other web scraping libraries.
Competitors
Some other Python libraries compete with Beautiful Soup, including:
- Scrapy
- Requests-HTML
- lxml
- Selenium
Each of these libraries has its strengths and weaknesses, so the best choice for a particular task will depend on the specific requirements of that task.
Here are some examples of how Beautiful Soup can be used:
- To extract the title of a web page
- To remove the links from a web page
- To extract the text from a web page
- To extract the data from a form
Python code samples
Here are some examples of Python code that can be used to interact with Beautiful Soup:
import requests
from bs4 import BeautifulSoup
# Get the HTML of a web page
url = 'https://www.example.com/'
response = requests.get(url)
# Create a Beautiful Soup object
soup = BeautifulSoup(response.content, 'html.parser')
# Extract the title of the web page
title = soup.title
# Extract the links from the web page
links = soup.find_all('a')
# Extract the text from the web page
text = soup.get_text()
# Extract the data from a form
form = soup.find_all('form')[0]
input_fields = form.find_all('input')
Beautiful Soup is a powerful and versatile library for web scraping. It is easy to use, versatile, and well-documented. Beautiful Soup can be used for many tasks and is a valuable tool for web scraping.