dict.get() takes no keyword arguments
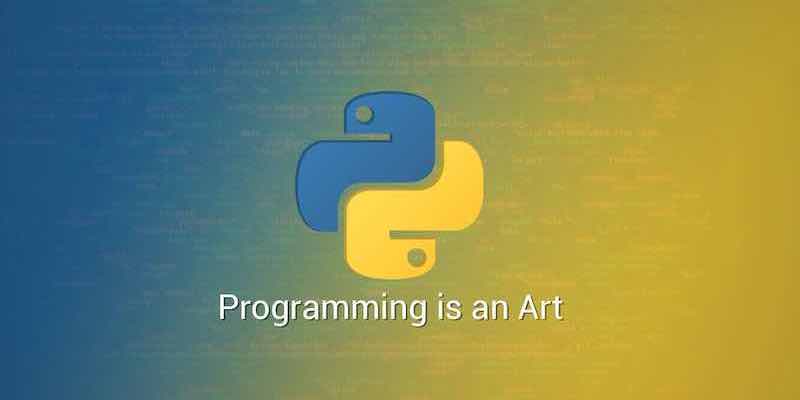
The error message “dict.get() takes no keyword arguments” occurs when you try to use keyword arguments while calling the get()
method on a dictionary in Python. The get()
method only takes positional arguments for the key and the default value.
Here is the correct usage and a demonstration of how you might inadvertently cause this error:
Correct Usage of dict.get()
:
The dict.get()
method can retrieve the value associated with a key in the dictionary. It takes two positional arguments: the key to look up and an optional default value to return if the key is not found. Here’s an example:
my_dict = {'a': 1, 'b': 2, 'c': 3}
# Using get() method to retrieve the value for key 'a'
value = my_dict.get('a')
print(value) # Output: 1
# Using get() method with a default value
value = my_dict.get('d', 4)
print(value) # Output: 4
Incorrect Usage Leading to the Error:
If you try to use keyword arguments while calling dict.get()
, you will get the error “dict.get() takes no keyword arguments”. Here’s an example:
my_dict = {'a': 1, 'b': 2, 'c': 3}
# Incorrect usage of get() method with keyword arguments
value = my_dict.get(key='a', default=4) # This will cause an error
How to Fix the Error:
To fix this error, you should use positional arguments instead of keyword arguments while calling dict.get()
. Here’s the corrected version of the above code:
my_dict = {'a': 1, 'b': 2, 'c': 3}
# Corrected usage of get() method with positional arguments
value = my_dict.get('a', 4)
print(value) # Output: 1
Decision:
The “dict.get() takes no keyword arguments” error is caused by using keyword arguments instead of positional arguments while calling the get()
Method on a dictionary. To avoid this error, use positional arguments for the key and the default value while using the get() method. Remembering the correct method signatures will help you avoid such mistakes and write more effective Python code.