Gensim: A Python library for unsupervised topic modeling
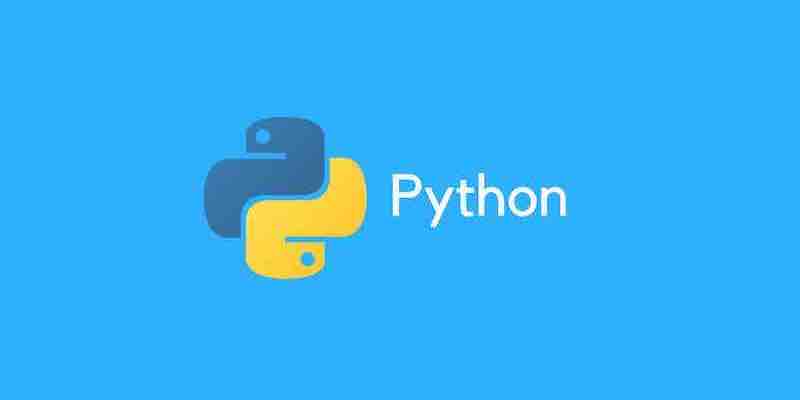
Using modern statistical machine learning, Gensim is a Python library for unsupervised topic modeling, document indexing, retrieval by similarity, and other natural language processing functionalities. Gensim is implemented in Python and Cython for performance.
Gensim was first released in 2009 by Radim Řehůřek. It was initially developed as a research project at the University of California, Berkeley. Gensim has since become a popular library for natural language processing and is used by a wide range of researchers and developers.
Benefits, Drawbacks
Gensim has many benefits, including:
- It is easy to use. Gensim provides a simple API that makes it easy to train and use topic models.
- It is efficient. Gensim uses Cython for performance to train large topic models on large corpora.
- It is versatile. Gensim can be used for a wide range of tasks, including topic modeling, document indexing, and retrieval by similarity.
Gensim also has a few drawbacks, including:
- It can be computationally expensive to train large topic models.
- It can be difficult to debug.
- It is not as well-documented as some other Python libraries.
Alternatives
Several other Python libraries compete with Gensim, including:
- spaCy
- NLTK
- TextBlob
- WordCloud
Each of these libraries has its strengths and weaknesses, so the best choice for a particular task will depend on the specific requirements of that task.
Usage samples
Here are some examples of how Gensim can be used:
- To train a topic model on a corpus of text
- To index a corpus of text for retrieval by similarity
- To retrieve documents that are similar to a given document
- To visualize a topic model
Python code samples
Here are some examples of Python code that can be used to interact with Gensim:
import gensim
# Create a corpus of text
corpus = gensim.corpora.BrownCorpus()
# Train a topic model on the corpus
model = gensim.models.ldamodel.LdaModel(corpus, num_topics=10)
# Index the corpus for retrieval by similarity
index = gensim.similarities.SparseMatrixSimilarity(corpus)
# Retrieve documents that are similar to a given document
document = "This is a document."
similar_documents = index.most_similar(document)
# Visualize the topic model
model.save('model.lda')
Gensim is a powerful and versatile library for unsupervised topic modeling. It is easy to use, efficient, and versatile. Gensim can be used for various tasks and is a valuable tool for natural language processing.