GREATEST() Function
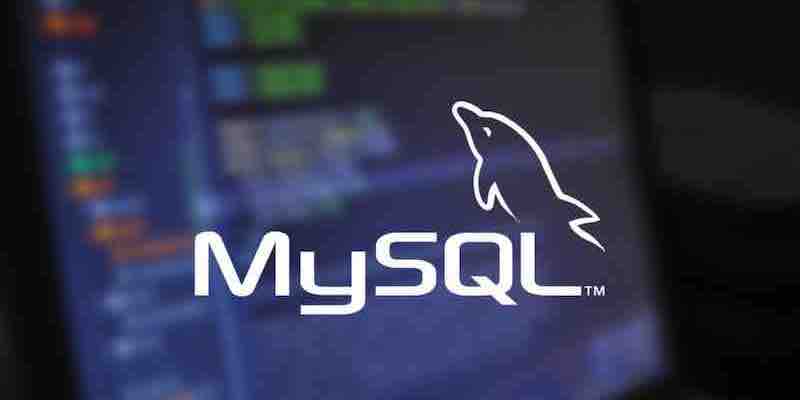
In MySQL, GREATEST()
is a function that returns the most significant value among the list of arguments provided. It compares values based on their data type and returns the greatest value.
GREATEST(value1, value2, ..., valueN)
Usage:
- Basic Usage:
SELECT GREATEST(3, 5, 7, 2);
This will return 7
because 7 is the largest value among the provided numbers.
- With Strings: When used with strings,
GREATEST()
Compares values based on their string values:
SELECT GREATEST('a', 'b', 'c');
This will return 'c'
.
- With Tables: Suppose you have a table named
products
with columnsprice1
,price2
, andprice3
. If you want to find the highest price among the three columns for each product, you can use:
SELECT product_name, GREATEST(price1, price2, price3) AS highest_price
FROM products;
- Handling NULL Values: If any of the values in the list is NULL, the
GREATEST()
function will return NULL unless all the values are NULL. To avoid this, you can use theCOALESCE()
Function to handle NULL values:
SELECT GREATEST(COALESCE(price1, 0), COALESCE(price2, 0), COALESCE(price3, 0)) AS highest_price
FROM products;
This will treat NULL values as 0 when determining the greatest price.
Note:
- The
GREATEST()
function is the opposite of theLEAST()
function, which returns the smallest value among the provided arguments. - If all arguments are NULL,
GREATEST()
it will return NULL. GREATEST()
Is a standard SQL function, and its behavior is consistent across many relational database systems, not just MySQL.
The GREATEST() Function in MySQL provides a convenient way to retrieve the largest value among a set of values, making it useful in various data analysis and transformation tasks.
GREATEST() And MAX()
Both GREATEST()
and MAX()
are functions in MySQL that deal with finding the maximum value, but they are used in different contexts and have distinct behaviors. Here are the key differences between GREATEST()
and MAX()
:
1. Context of Usage:
- GREATEST():
- It compares multiple values provided as arguments and returns the greatest value among them.
- It operates on a list of expressions within a single row.
SELECT GREATEST(3, 5, 7, 2); -- Returns 7
- MAX():
- It is an aggregate function that retrieves the maximum value from a column across multiple rows.
- It operates on a set of values in a column.
SELECT MAX(price) FROM products; -- Returns the highest price from the 'products' table
2. Handling NULL Values:
- GREATEST():
- If any of the arguments are NULL,
GREATEST()
will return NULL unless all the values are NULL.
SELECT GREATEST(3, NULL, 7); -- Returns NULL
- MAX():
- It ignores NULL values in the column and returns the maximum non-NULL value. If all values are NULL, then
MAX()
returns NULL.
SELECT MAX(price) FROM products; -- Returns the highest non-NULL price
3. Use with GROUP BY:
- GREATEST():
- It doesn’t directly interact with the
GROUP BY
clause since it operates on individual rows. - MAX():
- It is commonly used with the
GROUP BY
clause to find the maximum value for each group of rows.
SELECT category, MAX(price) AS highest_price
FROM products
GROUP BY category; -- Returns the highest price for each product category
4. Number of Arguments/Operands:
- GREATEST():
- It can take multiple arguments (two or more) and returns the greatest among them.
- MAX():
- It operates on a single column and returns the maximum value from that column.
While both GREATEST()
and MAX()
help in determining the maximum value; they serve different purposes. GREATEST() compares values within a single row, whereas MAX() is an aggregate function that finds the maximum value in a column across multiple rows.