Harnessing the Power of SciPy: Your Gateway to Scientific Computing in Python
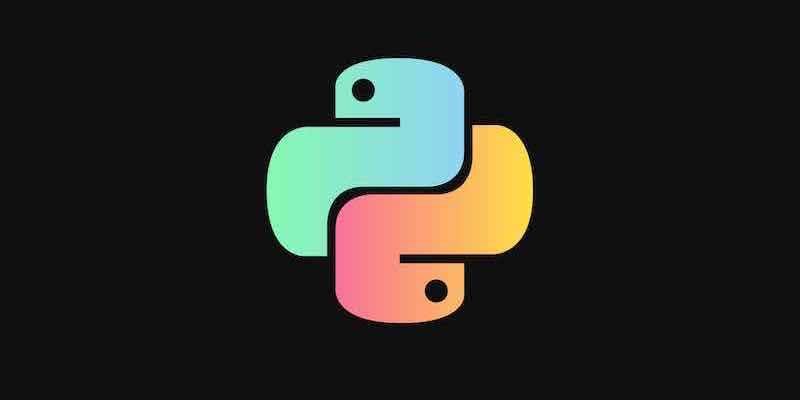
Python has emerged as a frontrunner thanks to its extensive library range that facilitates various scientific and technical computations in the dynamic world of data science and computational science. One such library that stands out is SciPy, a free and open-source Python library used for high-level analyses.
Introduction to SciPy
SciPy, built on the NumPy extension, is a library that offers tools and techniques for analysis in mathematics, science, and engineering. It is widely used for optimization, linear algebra, integration, interpolation, special functions, FFT, signal and image processing, ODE solvers, and other daily tasks in science and engineering.
Getting Started with SciPy
Before we dive into the functionalities of SciPy, it is essential to install the library. You can do this using the following command:
!pip install scipy
Linear Algebra with SciPy
SciPy provides many functions to work with linear algebra, one of the foundational aspects of data science. Here is a simple example demonstrating how to solve a linear equation using SciPy:
from scipy import linalg
import numpy as np
# Define the coefficient matrix A and the constant matrix B
A = np.array([[1, 2], [3, 4]])
B = np.array([[5], [6]])
# Solve the linear equation
x = linalg.solve(A, B)
print(x)
Optimization using SciPy
Optimization is a critical aspect of various scientific computations. SciPy offers functions to perform optimization in different scenarios. Here is a code snippet demonstrating optimization:
from scipy.optimize import minimize
def objective_function(x):
return x[0]*x[3]*(x[0]+x[1]+x[2])+x[2]
# Initial guesses
x0 = [1,5,5,1]
# Bounds [(min, max), ...]
b = (1.0,5.0)
bounds = (b, b, b, b)
# Solve optimization problem
solution = minimize(objective_function, x0, bounds=bounds)
print(solution)
Statistical Functions in SciPy
SciPy also offers a wide range of statistical functions. Here is a simple example demonstrating the use of statistical procedures in SciPy:
from scipy import stats
# Generate a random array
data = np.array([1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5, 5, 5])
# Calculate mean, median and mode
mean = np.mean(data)
median = np.median(data)
mode = stats.mode(data)
print(f"Mean: {mean}, Median: {median}, Mode: {mode.mode[0]}")
SciPy, with its extensive range of functionalities, serves as a powerful tool for scientists, engineers, and data analysts. Whether it’s solving complex linear equations, optimizing functions, or performing statistical analysis, SciPy has got you covered. We hope this blog post has given you a comprehensive insight into the capabilities of SciPy, encouraging you to explore and utilize this library to its fullest potential in your scientific computing endeavors.
Remember, the journey of mastering SciPy begins with a single step. Start experimenting with the code samples provided and venture into the fascinating scientific computing world with Python and SciPy.