Mastering the Art of Graceful Error Handling in Python
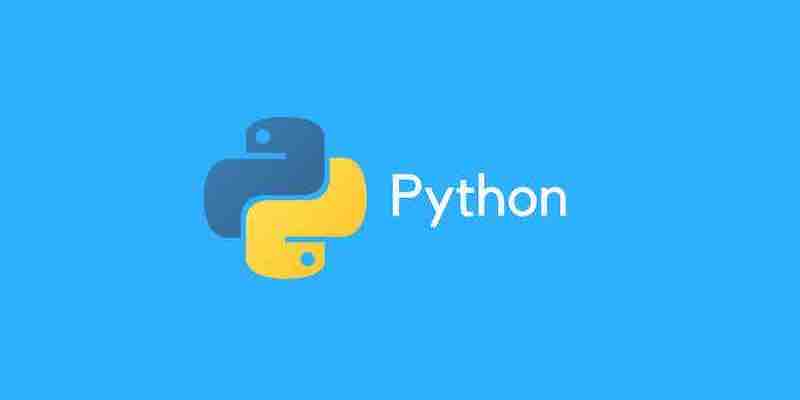
When embarking on your Python programming journey, it’s only a matter of time before you encounter errors or exceptions that halt your code’s execution. It’s not a matter of if but when. But fear not, as Python provides a sophisticated system to manage exceptions gracefully. We delve into the vital skill of Python exception handling, which enables developers to write robust programs capable of handling unforeseen errors gracefully.
Understanding Python Exceptions
Before diving into exception handling, it’s essential to understand what exceptions are. In Python, exceptions are events that disrupt the normal flow of a program. These could be errors like FileNotFoundError
when attempting to open a non-existent file or ValueError
when trying to convert an incompatible data type.
Python has many built-in exceptions, and understanding how to handle them adeptly is a hallmark of a seasoned Python developer.
The Try-Except Block
At the heart of Python exception handling is the try-except
block. Here’s a simplified breakdown of how it works:
- Try Block: The code segment where you anticipate an exception might occur.
- Except Block: The piece that defines how to handle the exception if it appears in the try block.
Here’s a basic example:
try:
result = 10 / 0
except ZeroDivisionError:
result = None
print("Error: Attempted to divide by zero.")
In this code snippet, the ZeroDivisionError
is caught in the except block, preventing the program from crashing and providing a user-friendly message instead.
Leveraging the Else and Finally Clauses
To further refine your error handling, you can use the else
and finally
Clauses:
- Else Block: This block executes if the try block does not raise any exceptions.
- Finally, Block: This code section will execute no matter what, ensuring that specific ‘cleanup’ actions are always performed.
try:
result = 10 / 2
except ZeroDivisionError:
result = None
print("Error: Attempted to divide by zero.")
else:
print("Division successful.")
finally:
print("Execution completed.")
In this example, since no error occurs, the else block executes, followed by the finally block, providing a structured approach to managing different outcomes.
Creating Custom Exceptions
As you progress in your Python journey, you may find the need to define your custom exceptions to handle specific error conditions unique to your program. Here’s how you can create a custom exception:
class CustomError(Exception):
pass
try:
raise CustomError("A custom error occurred")
except CustomError as e:
print(e)
In this instance, CustomError
is a user-defined exception class that inherits from the base Exception
class, allowing for more precise error handling.
Finale
Mastering Python exception handling allows developers to build resilient, robust programs capable of standing up to unexpected issues that might arise during execution. By utilizing try-except blocks, leveraging else and finally clauses, and even creating custom exceptions, you can ensure that your Python programs are robust but also user-friendly and resilient.
Remember, in the programming world, it’s not just about preventing errors but managing them gracefully when they inevitably occur. Happy coding!