Object of type datetime is not JSON serializable.
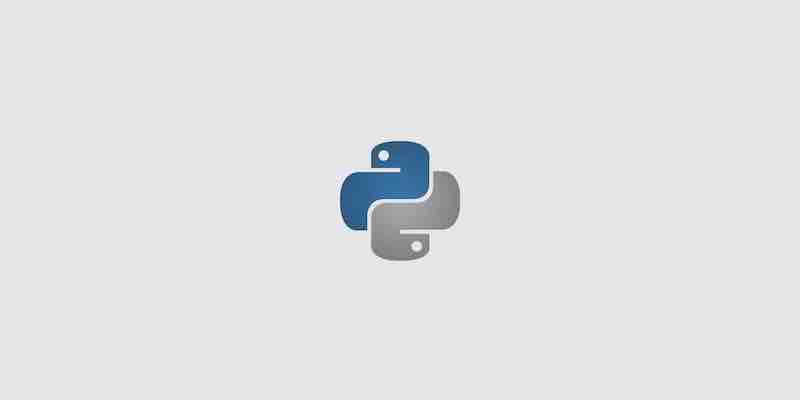
The error “Object of type datetime is not JSON serializable” occurs when you try to serialize a Python datetime
object into JSON format using the json
module. The json
module does not know how to convert a datetime
object into a JSON-compatible format by default.
To resolve this issue, you can use one of the following methods:
- Convert the
datetime
object to a string: Before serializing the object, you can convert thedatetime
object to a string using thestrftime
method.
import json
import datetime
dt = datetime.datetime.now()
dt_str = dt.strftime('%Y-%m-%d %H:%M:%S')
data = {'date': dt_str}
json_str = json.dumps(data)
- Use a custom JSON encoder: You can define a custom function to handle the
datetime
object during the serialization process.
import json
import datetime
class DateTimeEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, datetime.datetime):
return obj.isoformat()
return super(DateTimeEncoder, self).default(obj)
dt = datetime.datetime.now()
data = {'date': dt}
json_str = json.dumps(data, cls=DateTimeEncoder)
- Use a lambda function with
default
argument: Instead of creating a custom encoder class, you can use a lambda function with thedefault
argument of thejson.dumps
method.
import json
import datetime
dt = datetime.datetime.now()
json_str = json.dumps(data, default=lambda o: o.isoformat() if isinstance(o, datetime.datetime) else None)
Choose the method that best fits your needs. The first method is straightforward but requires you to convert each object manually. The second and third methods are more automated and can handle multiple datetime
objects in a data structure.