Python and CSV Files: A Powerful Combination
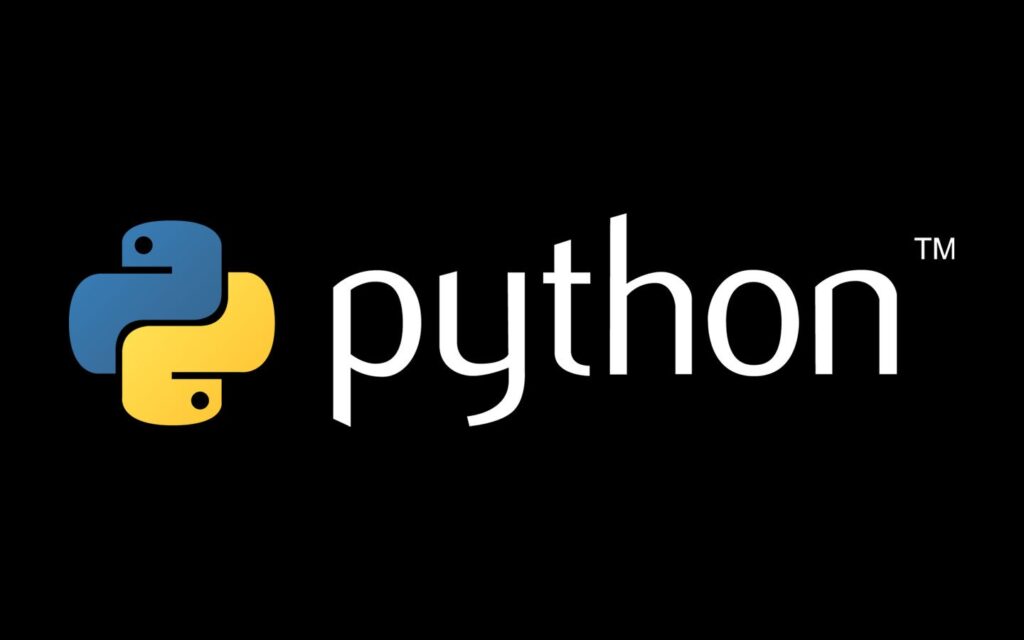
CSV files are a popular format for storing data. They are easy to read and write and can store various data. Python provides many libraries for processing CSV files, which makes it easy to read, write, and manipulate CSV data.
Popular Python Libraries for CSV Files
There are many popular Python libraries for processing CSV files. Some of the most popular libraries include:
- CSV: The CSV library is a built-in library for Python. It provides a simple interface for reading and writing CSV files.
- Pandas: The pandas’ library is a popular library for data analysis. It provides several functions for reading, writing, and manipulating CSV data.
- numpy: The numpy library is a popular library for scientific computing. It provides some functions for working with arrays, which can be used to process CSV data.
Code Samples for Processing CSV Files
Here are some code samples for processing CSV files using Python:
Python provides many libraries for processing CSV files. These libraries make reading, writing, and manipulating CSV data easy. Using Python, you can efficiently process CSV data to perform various tasks, such as data analysis, cleaning, and visualization.