Python’s Magic Methods: Operator Overloading
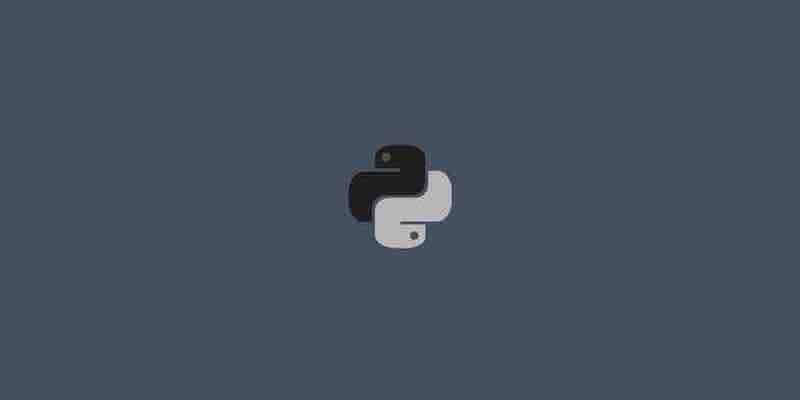
In the enchanting world of Python, there’s a touch of magic that allows developers to redefine or “overload” the default behavior of operators. This capability, known as operator overloading, is a manifestation of polymorphism, allowing objects of different types to be manipulated using the same operator syntax. Let’s journey to uncover the secrets of operator overloading in Python.
What is Operator Overloading?
Operator overloading lets developers change the meaning or implementation of existing operators for user-defined types. For instance, using the +
operator to add two objects of a custom class or the ==
operator to compare them based on custom criteria.
The Magic Behind the Scenes: Dunder Methods
The magic of operator overloading in Python is facilitated by unique methods, often called “dunder” (double underscore). These methods have names surrounded by double underscores, like __add__
or __lt__
.
Code Samples: Crafting a Custom Complex Number Class
Let’s create a custom ComplexNumber
class and overload some operators for it.
class ComplexNumber:
def __init__(self, real, imag):
self.real = real
self.imag = imag
# Overloading the `+` operator
def __add__(self, other):
return ComplexNumber(self.real + other.real, self.imag + other.imag)
# Overloading the `==` operator
def __eq__(self, other):
return self.real == other.real and self.imag == other.imag
# String representation for our custom class
def __str__(self):
return f"{self.real} + {self.imag}i"
# Testing our ComplexNumber class
num1 = ComplexNumber(3, 2)
num2 = ComplexNumber(1, 7)
print(num1 + num2) # Outputs: 4 + 9i
print(num1 == num2) # Outputs: False
More Magic Methods for Operator Overloading
__sub__
: Overloads-
__mul__
: Overloads*
__truediv__
: Overloads/
__floordiv__
: Overloads//
__mod__
: Overloads%
__pow__
: Overloads**
__lt__
,__le__
,__gt__
,__ge__
: Overload comparison operators<
,<=
,>
,>=
Respectively.
The Power of Polymorphism
Operator overloading is a testament to the power of polymorphism in Python. It allows developers to use familiar syntax while working with custom data types, leading to more readable and intuitive code.
With its magical dunder methods, operator overloading offers Python developers a way to infuse their custom objects with intuitive and familiar behaviors. It’s a tool that, when wielded with care, can make your code more expressive and more Pythonic. So, the next time you’re crafting a custom class, remember the magic of operator overloading and let your creativity run wild!