Redis: The Fast, In-Memory Database
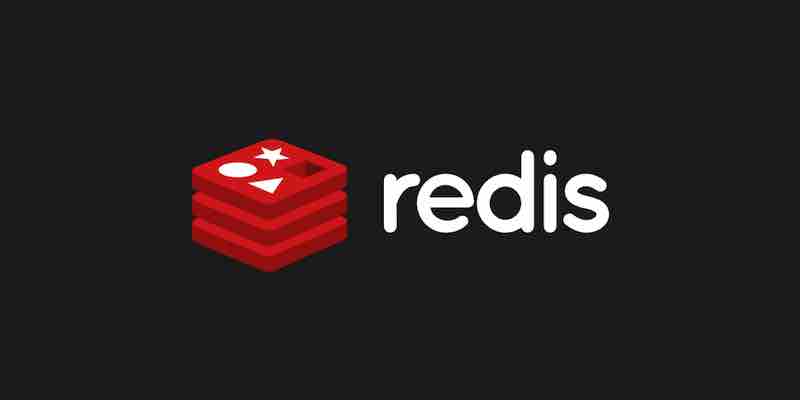
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It is famous for various applications, including web applications, real-time analytics, and gaming.
What is Redis?
Redis stands for REmote DIctionary Server. It is a key-value store that stores data in memory. This makes it very fast to retrieve data, as there is no need to read data from a disk. Redis also supports a variety of data structures, including strings, lists, sets, and hashes.
Benefits of Using Redis
There are many benefits to using Redis, including:
- Speed: Redis is swift for retrieving data and storing data in memory.
- Scalability: Redis is scalable and can be easily scaled up or down to meet the needs of your application.
- Flexibility: Redis supports a variety of data structures, which makes it flexible for a variety of applications.
- Ease of use: Redis is easy to use and has a simple API.
Drawbacks of Using Redis
There are a few drawbacks to using Redis, including:
- Data loss: If Redis loses power or crashes, all the data in memory will be lost.
- Security: Redis is less secure than other databases and can be vulnerable to attacks.
- Complexity: Redis can be complex to use, with a steep learning curve.
Competitors of Redis
There are a few other popular in-memory databases that compete with Redis, including:
- Memcached: Memcached is a more straightforward and less feature-rich database than Redis.
- Cassandra: Cassandra is a distributed database that is more scalable than Redis.
- MongoDB: MongoDB is a document-oriented database that is more flexible than Redis.
Usage Areas of Redis
Redis is used in a variety of applications, including:
- Web applications: Redis is often used as a web application cache to improve performance.
- Real-time analytics: Redis can store and analyze real-time data like user activity.
- Gaming: Redis is often used in gaming applications to store and manage game state data.
Python Libraries for Redis
Several Python libraries can be used to interact with Redis, including:
- redis: The official Python library for Redis.
- Redis-py-cluster: A library for working with Redis clusters.
- Redis-om: A library for working with Redis as a document store.
Python Code Examples
Here is some Python code that shows how to use Redis:
import redis
# Connect to Redis
r = redis.StrictRedis()
# Set a key-value pair
r.set("name", "Bard")
# Get the value of a key
name = r.get("name")
# Print the value
print(name)
Redis is a powerful and versatile in-memory database that can be used for various applications. It is fast, scalable, flexible, and has a simple API. Redis is a good option if you are looking for a fast and efficient database for your application.