Generating IDs via Sqids
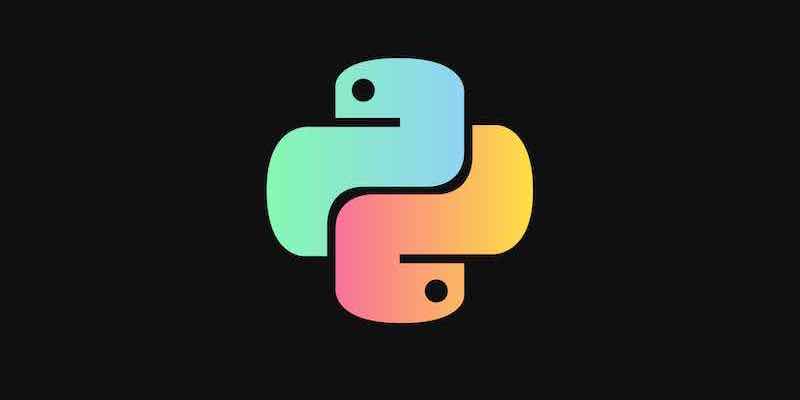
Sqids, formerly known as Hashids, is an open-source library designed to generate YouTube-like IDs from numbers. These IDs are concise, can be produced using a custom alphabet, and are guaranteed free from collisions.
Key Features and Information
Category | Description/Features |
---|---|
Visual Purpose | The primary use of Sqids is for visual appeal. It is ideal for those who prefer IDs over numbers in web applications. |
Use Cases | – Link shortening – Safe for URL usage – Event IDs – Quick collision-free ID generation – Fast lookups – Decoding back to numbers |
Not Suitable For | – Encrypting sensitive data (not an encryption library) – User IDs (can be decoded, revealing user count) |
Other Features | – Generate short IDs from non-negative numbers – Easy encoding and decoding – Auto-generated IDs free from common profanity – Custom IDs via shuffled alphabet – Randomized IDs for incremental numbers – Support in 40 programming languages – Consistent IDs across versions – Small library with a permissive license |
The main idea behind Sqids is to provide visually appealing IDs, especially for scenarios where you’d like to avoid displaying plain numbers. However, it’s essential to note that Sqids is not an encryption tool, so it’s not suitable for hiding sensitive data or user counts.
We can use Sqids in Python to produce IDs for a MySQL table. Sqids supports Python, allowing you to generate short, YouTube-like IDs from numbers. These IDs can then be used as unique identifiers in your MySQL table.
Steps to Use Sqids in Python for MySQL:
- Install the Sqids Library for Python:
You can install the Sqids library using pip:
pip install sqids
- Generate IDs Using Sqids:
After installing the library, you can use it to generate IDs. Here’s a basic example:
import sqids
# Initialize Sqids with a salt
sq = sqids.Sqids(salt="your_salt_here")
# Encode a number to get an ID
encoded_id = sq.encode(12345)
print(encoded_id) # This will give you a short ID
- Use the Generated ID in MySQL:
Once you have the encoded ID, you can use it as a unique identifier in your MySQL table. For instance, if you’re inserting a new record, you can use the generated ID as part of the insert statement.
import mysql.connector
# Connect to your MySQL database
conn = mysql.connector.connect(host="your_host", user="your_user", password="your_password", database="your_database")
cursor = conn.cursor()
# Insert data with the generated ID
query = "INSERT INTO your_table (id, data_column) VALUES (%s, %s)"
cursor.execute(query, (encoded_id, "your_data"))
conn.commit()
cursor.close()
conn.close()
- Decoding IDs:
If you need to decode the ID back to its original number, you can use thedecode
Method:
original_number = sq.decode(encoded_id)
print(original_number) # This will give you the original number (e.g., 12345)
Considerations:
- Ensure you use a unique salt when initializing Sqids. The salt ensures that the generated IDs are unique to your application.
- Sqids IDs are not encrypted; they are obfuscated. If you need to hide sensitive information, consider other methods or libraries.
- Ensure the generated ID does not collide with existing IDs in your MySQL table. While Sqids guarantees collision-free generation, it’s good practice to check for uniqueness in your database.
In summary, Sqids can be a helpful tool for generating visually appealing IDs in Python for a MySQL table, especially if you want to avoid displaying plain sequential numbers.