Are You Still Stuck in Monolithic Frontends? Why Micro Frontends are the Future of Web Development for Beginners
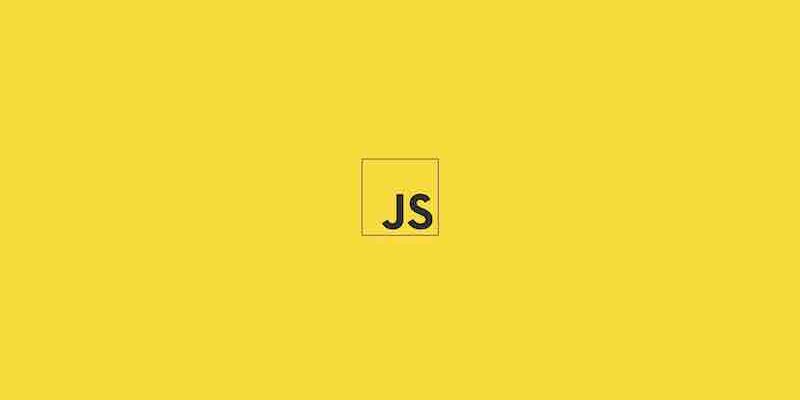
Micro frontend architecture is an approach to building web applications that decompose the user interface into independent, small, and reusable micro frontends. Each micro frontend is a self-contained module that can be developed, tested, deployed, and maintained independently by a separate team of developers. This allows for greater agility, scalability, and flexibility in developing large and complex web applications.
The micro frontend architecture is similar to the microservices architecture, where an extensive application is broken down into more minor, independent services that can be developed, deployed, and maintained independently. In the case of micro frontends, the focus is on breaking down the user interface into smaller, more manageable pieces that can be developed and maintained by separate teams.
Each micro frontend is responsible for a specific user interface area and communicates with other micro frontends through well-defined interfaces and APIs. This allows for greater modularity, reusability, and composability of the user interface, better isolation of failures, and easier debugging.
The micro frontend architecture can help organizations to build more scalable, maintainable, and modular web applications with improved development speed and flexibility.
A Sample Code
In this example, we have defined two React components: MyMicrofrontend and MyApp. MyMicrofrontend is a self-contained module that can be developed, tested, and deployed independently, and MyApp is the host application that integrates our micro frontend.
To use the micro frontend in our host application, we import the MyApp component and render it in our main application:
In this way, we can compose our user interface from multiple independent micro frontends, each of which can be developed and maintained separately.