Navmesh: A Pathfinding Tool for Python
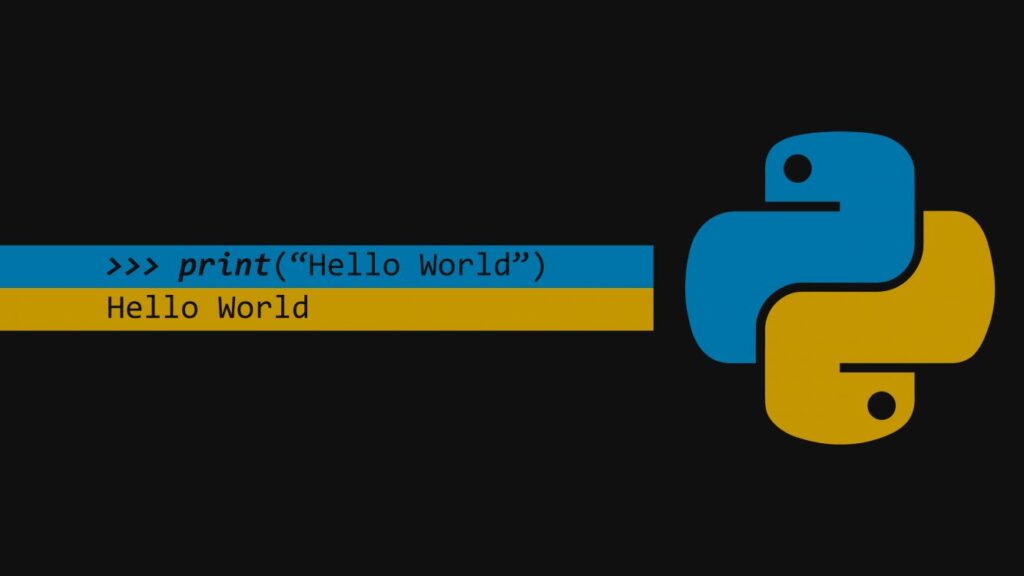
A navigation mesh, or Navmesh, is an abstract data structure used in artificial intelligence applications to aid agents in pathfinding through complicated spaces. This approach has been known since at least the mid-1980s in robotics, where it has been called a meadow map, and was popularized in video game AI in 2000.
A navigation mesh is a collection of two-dimensional convex polygons (a polygon mesh) that define which areas of an environment are traversable by agents. The polygons are typically created by “baking” the level geometry, which means that the polygons are made based on the walkable surfaces of the level.
Once a navigation mesh has been created, agents can find paths between different points in the environment. The pathfinding algorithm will typically start at the agent’s current location and search the navigation mesh for the shortest route to the desired destination.
Navigation meshes are a powerful tool for AI developers. They allow agents to navigate complex environments realistically and efficiently. Navigation meshes are used in various applications, including video games, robotics, and virtual reality.
Here are some of the benefits of using navigation meshes:
- They allow agents to navigate complex environments realistically and efficiently.
- They are relatively easy to create and maintain.
- They can be used in a wide variety of applications.
Here are some of the challenges of using navigation meshes:
- They can be computationally expensive to create and update.
- They can be challenging to scale to large environments.
- They can be difficult to use in dynamic environments.
Navigation meshes are a powerful tool for AI developers. They allow agents to navigate complex environments realistically and efficiently. Navigation meshes are used in various applications, including video games, robotics, and virtual reality.
How to Use Navmesh to Create Realistic and Efficient Pathfinding for Your Agents
Navmesh is a pathfinding tool for Python that allows you to create realistic and efficient pathfinding for your agents. Navmesh is a collection of two-dimensional convex polygons (a polygon mesh) that define which areas of an environment are traversable by agents. The polygons are typically created by “baking” the level geometry, which means that the polygons are made based on the walkable surfaces of the level.
Once a navigation mesh has been created, agents can find paths between different points in the environment. The pathfinding algorithm will typically start at the agent’s current location and search the navigation mesh for the shortest route to the desired destination.
Benefits of Using Navmesh
There are many benefits to using Navmesh for pathfinding in Python. Navmesh is a powerful tool for creating realistic and efficient pathfinding for your agents. Navmesh is also relatively easy to use and maintain.
Here are some of the benefits of using Navmesh:
- Realistic pathfinding: Navmesh allows you to create realistic pathfinding for your agents. This is because Navmesh considers the actual geometry of the environment when finding paths. This results in agents that can navigate complex environments naturally and realistically.
- Efficient pathfinding: Navmesh is also an efficient pathfinding tool. This is because Navmesh uses various techniques to optimize the pathfinding process. This results in agents that can find paths quickly and efficiently.
- Easy to use and maintain: Navmesh is also relatively easy to use and maintain. This is because Navmesh is a well-documented and well-supported tool. There are also a variety of resources available to help you learn how to use Navmesh.
Related Python Frameworks
Many Python frameworks can be used with Navmesh. Some of the most popular frameworks include:
- Pygame: Pygame is a popular Python framework for game development. Pygame includes a built-in Navmesh class that can be used to create and use navigation meshes.
- Panda3D: Panda3D is another popular Python framework for game development. Panda3D also includes a built-in Navmesh class that can be used to create and use navigation meshes.
- Unity: Unity is a popular game engine that supports Python scripting. Unity also includes a built-in Navmesh class that can be used to create and use navigation meshes.
Alternatives to Navmesh
Several alternatives to Navmesh can be used for pathfinding in Python. Some of the most popular options include:
- A:* A* is a well-known pathfinding algorithm that can find paths in various environments. A* is a relatively simple algorithm, but it can sometimes be slow.
- Dijkstra’s algorithm: Dijkstra’s algorithm is another well-known pathfinding algorithm that can find paths in various environments. Dijkstra’s algorithm is generally faster than A* but can be more challenging to implement.
- RRT: RRT is a recent pathfinding algorithm that can find paths in complex environments. RRT is generally faster than A* and Dijkstra’s algorithm but can be more challenging.
Sample Code
Here is a sample code that shows how to use Navmesh to find a path between two points in a Python game:
This code will create a Navmesh object, load a Navmesh from a file, create a start and end point, find the path between the beginning and end points, and draw the way on the screen.