Not enough segments | Python
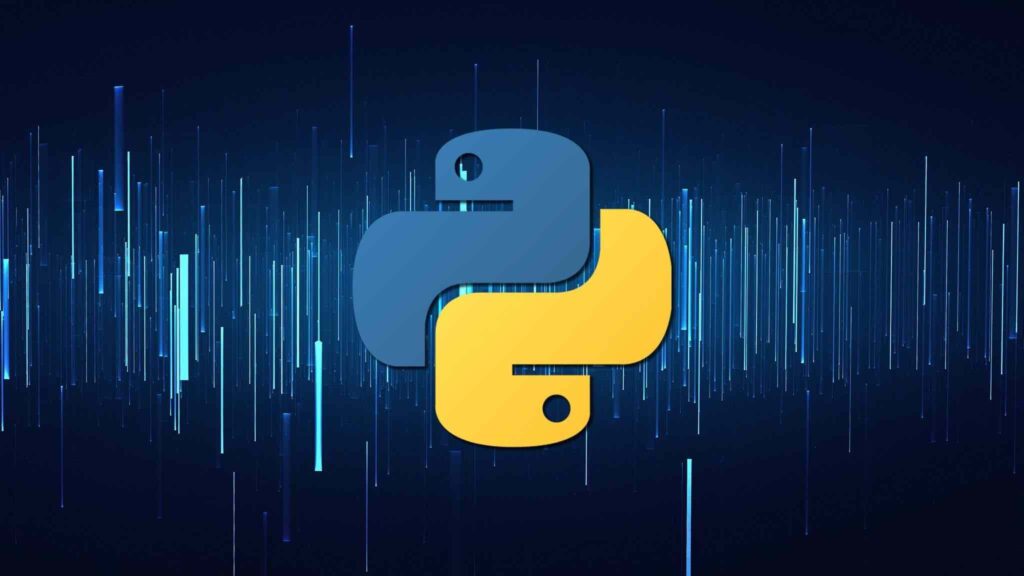
The error message “Not enough segments” typically occurs when you are working with a string that is expected to have a certain number of segments separated by a delimiter, but the string does not have enough segments to satisfy the requirement.
For example, suppose you have a string that represents a date in the format “YYYY-MM-DD”, and you try to split the string using the hyphen (-) as a delimiter, like this:
date_str = “2022-03”
year, month, day = date_str.split(“-“)
In this example, date_str only contains the year and month segments, but we are trying to split it into three segments using the hyphen as a delimiter. When the code is executed, Python raises the “Not enough segments” error because there are not enough segments in date_str to satisfy the assignment of three variables.
To fix this error, you must ensure that the string you are working with has the correct number of segments. In the above example, you could add a default day value to the date_str string to ensure that it always has three segments:
date_str = “2022-03”
year, month, day = date_str.split(“-“) + [“01”]
In this updated code, we use the + operator to concatenate a default day value of “01” to the split() method result. This ensures that date_str always has three segments, even if the original string is missing the day segment.