Pandas Unveiled: Your Guide to Data Analysis in Python
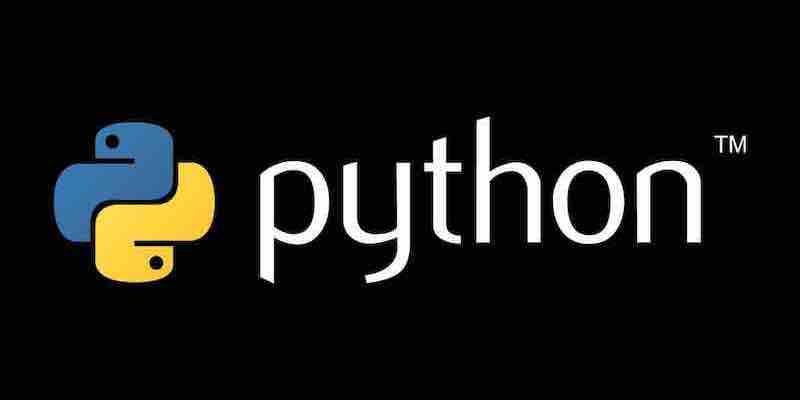
In data science and analysis, the ability to manipulate and analyze data efficiently is a skill that cannot be overstated. One tool that has revolutionized data handling is Pandas, a high-level data manipulation tool developed by Wes McKinney. It is built on the Numpy package, and its vital data structure is called DataFrame, which you can think of as an in-memory 2D table (like a spreadsheet) with labeled axes (rows and columns) but can also be used to represent higher-dimensional data. In this blog post, we will explore the functionalities of Pandas, accompanied by code samples, to help you start your data analysis journey.
Introduction to Pandas
Pandas is an open-source data analysis and manipulation tool that provides flexible data structures that make data manipulation in Python easy and efficient. It’s a must-have tool for any data scientist or data analyst with Python.
Getting Started with Pandas
To begin your journey with Pandas, you first need to install the library using the following command:
!pip install pandas
Once installed, you can import the library and start using it as shown below:
import pandas as pd
Creating and Reading DataFrames
DataFrames are the primary data structure in Pandas. You can create a data frame from scratch or read data from various file formats such as CSV, Excel, JSON, etc. Here are some examples:
# Creating a DataFrame from scratch
data = {'Name': ['John', 'Anna', 'Peter'], 'Age': [28, 22, 35], 'City': ['New York', 'Paris', 'London']}
df = pd.DataFrame(data)
print(df)
# Reading data from a CSV file
df = pd.read_csv('path/to/your/csvfile.csv')
Data Manipulation with Pandas
Pandas offers a wide range of data manipulation operations. Here are some everyday operations:
# Selecting a column
df['Name']
# Selecting multiple columns
df[['Name', 'City']]
# Selecting rows
df.iloc[0] # Selects the first row
# Filtering data
df[df['Age'] > 25]
# Adding a new column
df['Country'] = ['USA', 'France', 'UK']
# Dropping a column
df.drop('Country', axis=1, inplace=True)
Data Analysis with Pandas
Pandas also provide functions to perform data analysis. Here are some examples:
# Descriptive statistics
df.describe()
# Finding the mean
df['Age'].mean()
# Finding the median
df['Age'].median()
# Finding the correlation
df.corr()
Data Visualization with Pandas
Pandas integrates with Matplotlib to provide straightforward data visualization. Here’s how you can plot data using Pandas:
import matplotlib.pyplot as plt
# Line plot
df.plot(x='Name', y='Age', kind='line')
plt.show()
# Bar plot
df.plot(x='Name', y='Age', kind='bar')
plt.show()
Pandas is a robust tool for anyone looking to analyze and manipulate data efficiently in Python. Its wide range of functionalities allows easy data cleaning, manipulation, and analysis. We hope this blog post serves as a stepping stone in your journey to mastering data analysis with Pandas. Remember, the best way to learn is by doing. So, start experimenting with these code samples and explore the vast functionalities that Pandas has to offer.
Happy Coding!