PyLint: A Python Static Code Analyzer
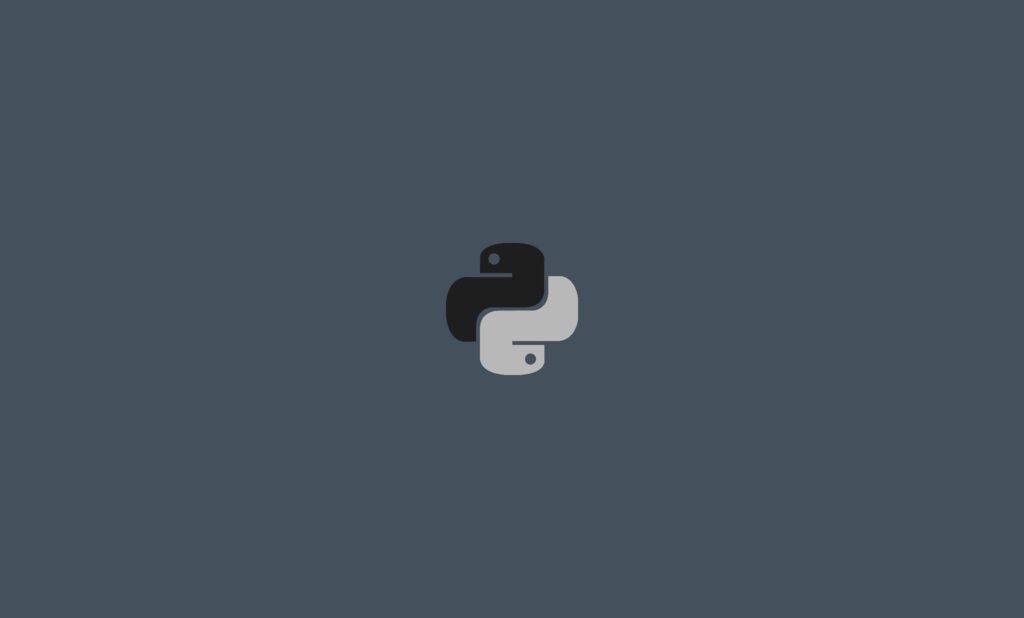
PyLint is a Python static code analysis tool that helps you find potential bugs, improve code quality, and enforce coding standards. It is a free and open-source tool that can be used on any Python project.
How PyLint Works
PyLint analyzes your code without actually running it. It looks for potential problems in your code, such as:
- Syntax errors: PyLint can detect syntax errors in your code. This can help you to avoid mistakes when you run your code.
- Potential bugs: PyLint can identify possible bugs in your code. This can help you to fix bugs before they cause problems.
- Coding standards: PyLint can check your code for compliance with coding standards. This can help you to write code that is consistent and easy to read.
Using PyLint
PyLint is a command-line tool. To use PyLint, you need to install it first. Once you have installed PyLint, you can run it on your code by typing the following command:
Code snippet
pylint my_code.py
PyLint will then analyze your code and print out a report of any potential problems it finds.
Benefits of Using PyLint
There are many benefits to using PyLint, including:
- Improved code quality: PyLint can help you improve your code quality by finding potential bugs and enforcing coding standards.
- Reduced development time: PyLint can help you to reduce development time by finding bugs early in the development process.
- Improved team collaboration: PyLint can help enhance team collaboration by ensuring everyone follows the same coding standards.
PyLint is a powerful tool that can help you improve your Python code’s quality. It is a free and open-source tool that can be used on any Python project. If you are serious about writing high-quality Python code, use PyLint.
Here are some additional details about PyLint:
- PyLint is a Python library that can be used from the command line or as part of an IDE.
- PyLint is highly configurable, so you can customize it to meet your needs.
- PyLint is supported by a large community of users and developers.
- PyLint is a valuable tool for any Python developer who wants to write high-quality code.
Code Samples
Here are some code samples that demonstrate how to use PyLint:
# This code has a syntax error.
def foo():
print("Hello, world!")
# This code has a potential bug.
def bar():
if x == 1:
return 1
else:
return 2
# This code does not follow the coding standards.
def baz():
print("Hello, world!")
pylint foo.py
pylint bar.py
pylint baz.py
The output of PyLint will be similar to the following:
Code snippet
foo.py:1:1: E1101: Missing docstring
foo.py:3:8: E722: Possible missing return statement
bar.py:2:12: E722: Possible missing return statement
baz.py:1:1: E1101: Missing docstring
These errors can be fixed by making the necessary changes to the code.