PyScript: A Gateway to Seamless Scripting and Automation
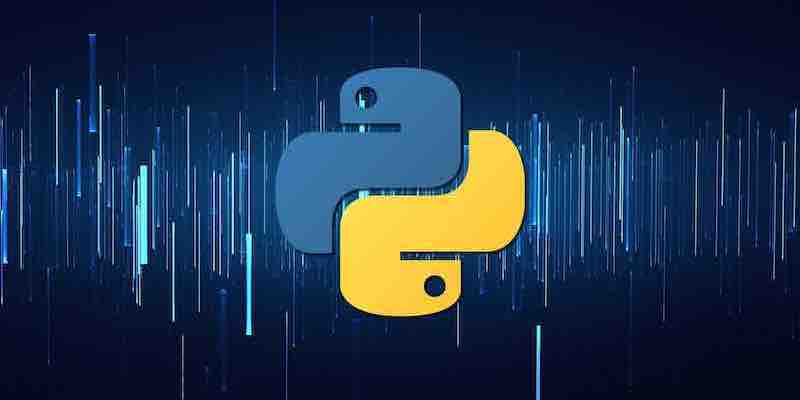
Python has carved a niche for itself in the dynamic programming landscape due to its simplicity and versatility. Among Python’s various tools and libraries, PyScript is a robust scripting environment that facilitates automation and streamlines multiple tasks. We will explore the functionalities of PyScript, accompanied by illustrative examples, to get you started on your PyScript journey.
PyScript is a Python package that allows users to execute Python scripts seamlessly. It is particularly beneficial for automating repetitive tasks, data processing, and integrating different software components. Before we delve into examples, let’s understand how to set up and configure PyScript in your Python environment.
Setting Up PyScript
To begin with, you need to install the MyScript package. You can do this using the following pip command:
pip install pyscript
Ensure you have Python installed in your system before running the above command.
Writing Your First PyScript
Once the installation is complete, you can start writing Python scripts using PyScript. Here is a simple script that prints a greeting message:
# greeting.py
from pyscript import PyScript
@PyScript
def greeting(name):
return f"Hello, {name}!"
if __name__ == "__main__":
print(greeting("World"))
To run this script, save it as greeting.py
Execute it in your terminal:
python greeting.py
You should see the output:
Hello, World!
Automating Tasks with PyScript
PyScript shines when it comes to automating repetitive tasks. Let’s consider a script that automates the process of creating a directory and moving files into it:
import os
from pyscript import PyScript
@PyScript
def organize_files(directory, file_extension, target_folder):
if not os.path.exists(target_folder):
os.makedirs(target_folder)
for file_name in os.listdir(directory):
if file_name.endswith(file_extension):
os.rename(os.path.join(directory, file_name), os.path.join(target_folder, file_name))
if __name__ == "__main__":
organize_files('./', '.txt', './text_files')
In this script, we define a function organize_files
That takes a directory path, a file extension, and a target folder name as inputs. It then moves all files with the specified extension into the target folder.
Leveraging PyScript for Data Processing
PyScript can also be utilized for data processing tasks. Here’s an example where we use PyScript to read a CSV file and perform some fundamental data analysis:
import pandas as pd
from pyscript import PyScript
@PyScript
def data_analysis(csv_file):
data = pd.read_csv(csv_file)
print(data.describe())
if __name__ == "__main__":
data_analysis('data.csv')
In this script, we use the pandas library to read a CSV file and print some basic statistics about the data.
PyScript is a powerful tool in the Python ecosystem, facilitating seamless scripting and automation. Whether automating mundane tasks or performing data analysis, PyScript can be valuable in your Python toolkit. As we have seen in the examples above, it integrates smoothly with Python, allowing for efficient and streamlined scripting solutions.