Rediscovering Data: A Dive into Redis with Python
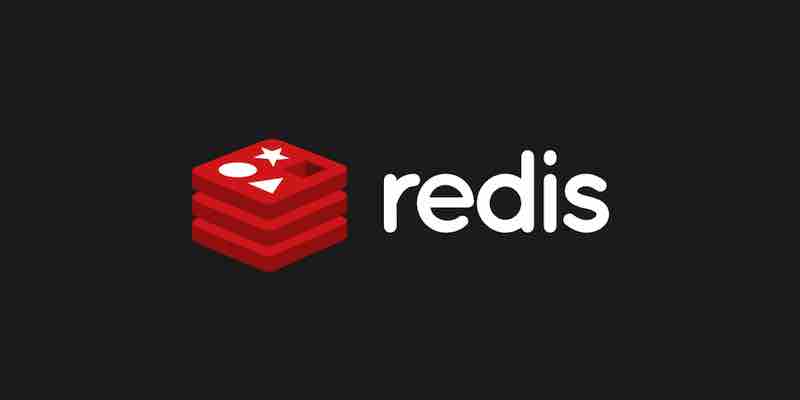
Redis, an in-memory data structure store, is often used as a database, cache, and message broker. Its versatility and speed make it a popular choice among developers. The possibilities are endless when combined with Python, one of the most widely-used programming languages. In this article, we’ll explore the basics of Redis and its integration with Python and provide some hands-on code samples.
1. Introduction to Redis
Redis stands for “REmote DIctionary Server.” It supports various data structures such as strings, hashes, lists, and more. Its in-memory nature ensures high performance, making it suitable for scenarios where high-speed access to data is crucial.
2. Setting Up Redis
Before diving into Python, ensure you have Redis installed. You can download and install Redis from the official website.
3. Integrating Redis with Python
To interact with Redis using Python, you’ll need the redis
library. Install it using pip:
pip install redis
4. Python Code Samples
a. Connecting to Redis
import redis
client = redis.StrictRedis(host='localhost', port=6379, db=0)
b. Setting and Getting a Key
client.set('hello', 'world')
print(client.get('hello')) # Outputs: b'world'
c. Working with Lists
client.lpush('fruits', 'apple', 'banana', 'cherry')
print(client.lrange('fruits', 0, -1)) # Outputs: [b'cherry', b'banana', b'apple']
d. Working with Hashes
client.hset('user:1', 'name', 'John Doe')
client.hset('user:1', 'age', '30')
print(client.hgetall('user:1')) # Outputs: {b'name': b'John Doe', b'age': b'30'}
e. Setting a Key with Expiry
client.setex('session', 30, 'active') # Key will expire after 30 seconds
With its high-speed performance and versatility, Redis is a valuable tool in a developer’s arsenal. When paired with Python, it offers a seamless experience for data storage and retrieval. Whether you’re building a high-traffic website or a real-time analytics application, Redis and Python can supercharge your project.