AWS ECS: A Scalable and Managed Container Service
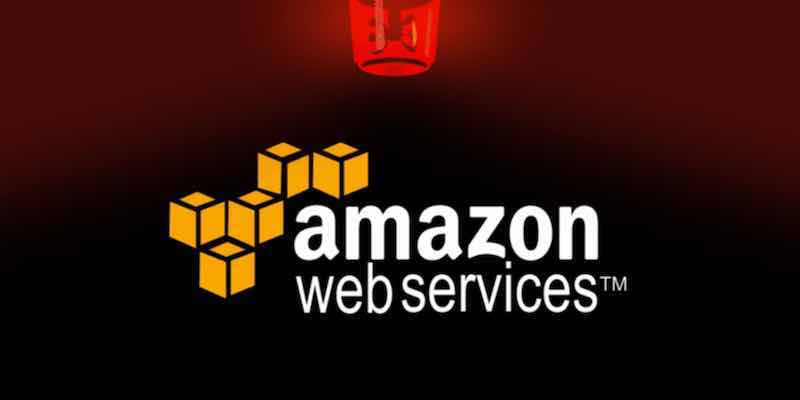
Run your containers on AWS with ease.
Amazon Elastic Container Service (ECS) is a scalable and managed container service that makes running Docker containers on AWS easy. ECS provides a high-level abstraction for managing containerized applications, so you can focus on building your applications and not managing the underlying infrastructure.
Benefits of using ECS
There are many benefits to using ECS, including:
- Scalability: ECS is highly scalable. You can quickly scale your containerized applications up or down based on demand.
- Reliability: ECS is a reliable service. Your containerized applications are replicated across multiple Availability Zones, so they are protected against data loss and downtime.
- Ease of use: ECS is easy to use. You can use the AWS Management Console, the AWS CLI, or the AWS SDKs to manage your containerized applications.
- Cost-effectiveness: ECS is a cost-effective way to run containerized applications. You only pay for the resources you use, so you can save money by optimizing your usage.
How to use ECS
To use ECS, you first need to create an ECS cluster. A cluster is a group of EC2 instances used to run your containerized applications. You can create a cluster using the AWS Management Console, the AWS CLI, or the AWS SDKs.
Once you have created a cluster, you can then deploy your containerized applications to the cluster. You can do this using the AWS Management Console, the AWS CLI, or the AWS SDKs.
Python libraries for ECS
There are several Python libraries that you can use to interact with ECS. These libraries can help you to:
- Create and manage ECS clusters
- Deploy containerized applications to ECS clusters
- Manage containerized applications on ECS clusters
Some of the most popular Python libraries for ECS include:
boto3
: The official AWS SDK for Pythonecs
: A Python library for interacting with ECSbotocore
: The core library for the AWS SDK for Python
Python code samples
Here is a simple Python code sample that shows how to create an ECS cluster:
import boto3
client = boto3.client('ecs')
cluster = client.create_cluster(
ClusterName='my-cluster'
)
print(cluster)
Here is a simple Python code sample that shows how to deploy a containerized application to an ECS cluster:
import boto3
client = boto3.client('ecs')
cluster = 'my-cluster'
task_definition = 'my-task-definition'
client.run_task(
Cluster=cluster,
TaskDefinition=task_definition
)
CLI samples
Here is a simple CLI command that shows how to create an ECS cluster:
aws ecs create-cluster --cluster-name my-cluster
Here is a simple CLI command that shows how to deploy a containerized application to an ECS cluster:
aws ecs run-task --cluster my-cluster --task-definition my-task-definition
ECS is a powerful container service that can help you deploy and manage your containerized applications on AWS. ECS provides a high-level abstraction for managing containerized applications, so you can focus on building your applications and not managing the underlying infrastructure.