Break in Python: A Powerful Tool for Loop Control
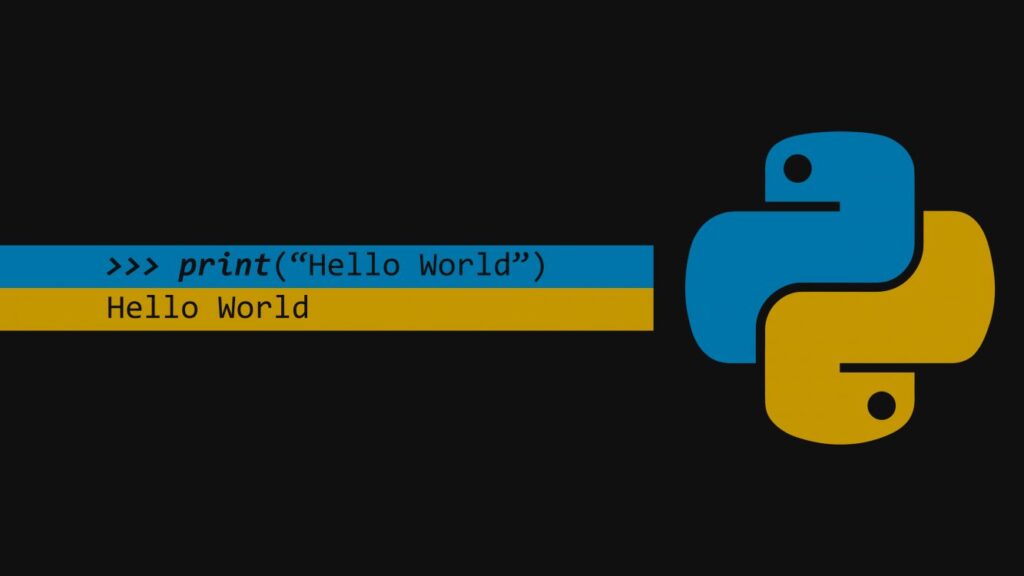
The break statement in Python can be used to exit a loop, either a for loop or a while loop. This can be useful for various reasons, such as when you want to stop iterating through a list if you find the value you’re looking for or to stop executing a loop if a specific condition is met.
Using Break with Nested For Loops
You can use the break statement to exit both loops simultaneously when you have nested for loops. For example, the following code will print the numbers from 1 to 10, but it will stop iterating through the outer loop if the number is divisible by 5:
for i in range(1, 11):
if i % 5 == 0:
break
print(i)
Break if Condition Met Example
You can also use the break statement with a condition. For example, the following code will print the numbers from 1 to 10, but it will stop iterating through the loop if the number is equal to 5:
for i in range(1, 11):
if i == 5:
break
print(i)
Conclusion
A break statement is a powerful tool that can be used to exit loops in Python. It can be used both for loops and while loops, with or without a condition. You can write more efficient and effective code by understanding how to use the break statement.
Here are some additional details about the break statement:
- The break statement can exit any type of loop, including “for” loops, “while” loops, and nested loops.
- The break statement can be used with or without a condition. The break statement will exit the current loop if no need is specified. If a condition is determined, the break statement will only leave the loop if the state is True.
- The break statement can be used to exit multiple nested loops at once.