Calculating The Least Common Multiple, LCM for MySQL
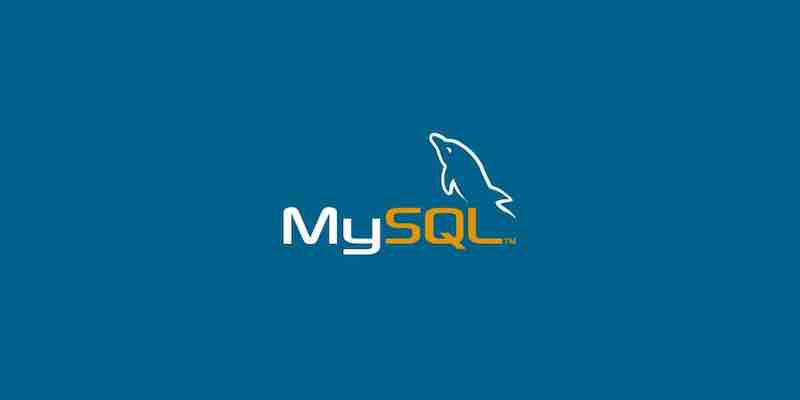
To calculate the smallest common multiple (often referred to as the Least Common Multiple or LCM) of two numbers in MySQL, you can use the formula:
[ \text{LCM}(a, b) = \frac{|a \times b|}{\text{GCD}(a, b)} ]
Where GCD is the Greatest Common Divisor of the two numbers.
MySQL doesn’t have a built-in function for GCD or LCM, but you can calculate the GCD using the Euclidean algorithm. Once you have the GCD, you can then calculate the LCM.
Here’s a stored procedure to calculate the LCM of two numbers:
DELIMITER //
CREATE FUNCTION GCD(a INT, b INT) RETURNS INT
DETERMINISTIC
BEGIN
DECLARE temp INT;
WHILE b != 0 DO
SET temp = a;
SET a = b;
SET b = temp % b;
END WHILE;
RETURN a;
END //
CREATE FUNCTION LCM(a INT, b INT) RETURNS INT
BEGIN
RETURN (a * b) / GCD(a, b);
END //
DELIMITER ;
MySQL requires the DETERMINISTIC
Keyword for stored functions to indicate that the function always produces the same result for the same input parameters.
After creating these functions, you can calculate the LCM of two numbers, say 12 and 15, as follows:
SELECT LCM(12, 15);
This will return 60
, which is the least common multiple of 12 and 15.