Demystifying Practical Cryptography: Python and MySQL in Focus
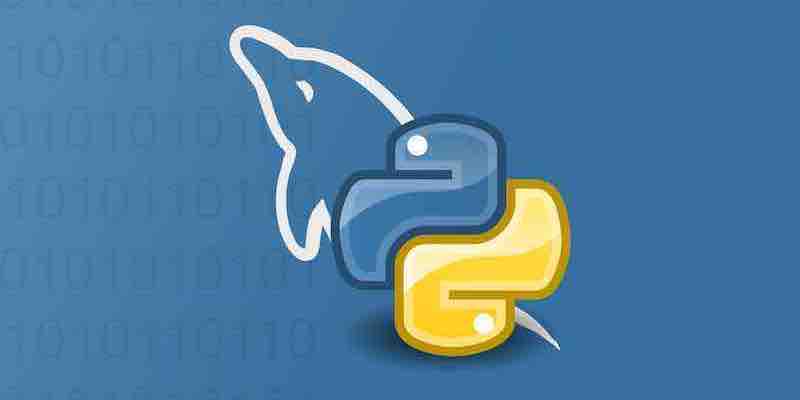
Theoretical Foundations of Cryptography: From MD5 to SHA-2
Understanding Cryptography
Cryptography is the art of secure communication. It involves transforming data to prevent unauthorized access. Over the years, various algorithms have emerged. Let’s delve into a few popular ones.
MD5: An Overview
Message Digest Algorithm 5 (MD5) was once a favorite. It produces a 128-bit hash from any input. However, its security vulnerabilities became evident over time. Today, it’s considered weak. Avoid MD5 for security-critical applications.
SHA Family: The Evolution
Secure Hash Algorithms (SHA) are cryptographic hash functions. They transform data into a fixed-size value. These values are fingerprints of the original data.
SHA-1: Not Without Its Flaws
SHA-1 is an improvement over MD5. It produces a 160-bit hash. Yet, vulnerabilities emerged. Experts now advise against using SHA-1 for cryptographic purposes.
SHA-2: The Current Gold Standard
SHA-2 is a family of hash functions. It includes SHA-224, SHA-256, SHA-384, SHA-512, SHA-512/224, and SHA-512/256. Of these, SHA-256 is widely used. It’s more secure than its predecessors.
Cryptography in Python: Libraries and Practical Code
Python’s Crypto Libraries
Python offers powerful libraries for cryptography. ‘PyCrypto’ and ‘cryptography’ are popular choices. They provide tools to implement secure systems.
Getting Started with ‘Cryptography’
First, install the library:
pip install cryptography
Symmetric Encryption with Fernet
Fernet guarantees the encrypted message won’t be tampered with. Here’s a simple encryption and decryption example using Fernet:
from cryptography.fernet import Fernet
# Generate a key
key = Fernet.generate_key()
cipher = Fernet(key)
# Encrypt a message
text = "Hello, World!"
encrypted_text = cipher.encrypt(text.encode())
print(encrypted_text)
# Decrypt the message
decrypted_text = cipher.decrypt(encrypted_text).decode()
print(decrypted_text)
Hashing with SHA-256
Python’s hashlib
module supports SHA-2, including SHA-256:
import hashlib
data = "Hello, World!"
hashed_data = hashlib.sha256(data.encode()).hexdigest()
print(hashed_data)
Cryptography in MySQL: Secure Your Database
MySQL and Cryptography
MySQL supports various cryptographic functions. These functions enhance data security.
Using MD5 and SHA in MySQL
Though we’ve highlighted their vulnerabilities, MD5 and SHA-1 are available in MySQL:
SELECT MD5('Hello, World!');
SELECT SHA1('Hello, World!');
Leveraging SHA-2 in MySQL
SHA-2 offers better security. MySQL supports it with the SHA2()
Function:
SELECT SHA2('Hello, World!', 256); -- For SHA-256
Cryptography Usage Areas in MySQL
- Password Storage: Always hash passwords before storing. Use salts for added security.
- Sensitive Data: Encrypt sensitive data like credit card numbers. Ensure decryption keys are secure.
- Data Integrity: Use hashes to verify data integrity. It ensures data hasn’t been tampered with.
Best Practices
Avoid using deprecated algorithms like MD5 and SHA-1. Always lean towards modern, proven cryptographic methods. When in doubt, consult security documentation or experts.
Cryptography is crucial in today’s digital age. Python and MySQL offer tools to build secure systems. Always be informed. Keep updating your knowledge. The security landscape evolves rapidly. Ensure your systems stay ahead of potential threats.