Design Patterns: A Guide to Reusable Software Solutions
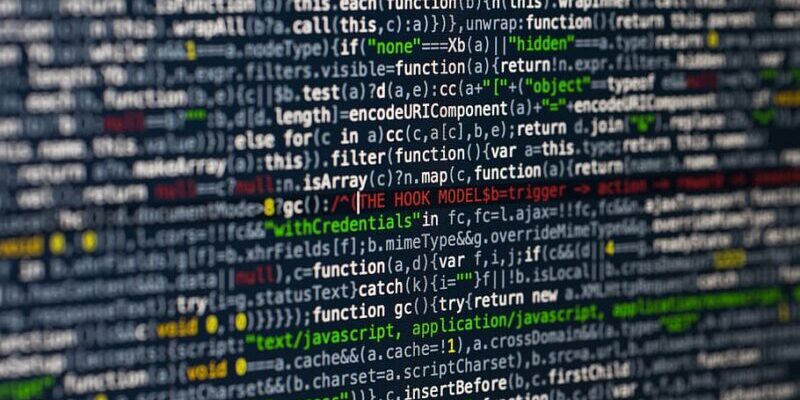
Learn how to use design patterns to write better, more maintainable code
Design patterns are reusable solutions to common software design problems. They provide a shared vocabulary for discussing design problems and answers and can help you write better, more maintainable code.
What are Design Patterns?
A design pattern is a general reusable solution to a commonly occurring problem in software design. A design pattern describes the problem, its solution, and the consequences of using the key. Design patterns are often used in object-oriented programming but can be applied to any programming language.
Why are Design Patterns Important?
Design patterns are essential for several reasons. They can help you to:
- Write better code: Design patterns can help you write more readable, understandable, and maintainable code.
- Solve everyday problems: Design patterns provide a shared vocabulary for discussing design problems and solutions. This can help you communicate with other developers more effectively and find solutions to problems you have not encountered before.
- Improve code quality: Design patterns can help you improve your code’s quality by making it more reusable, flexible, and extensible.
The History of Design Patterns
The Gang of Four (GoF) introduced the concept of design patterns in their book Design Patterns: Elements of Reusable Object-Oriented Software. The GoF identified 23 design patterns, which they divided into three categories: creational patterns, structural patterns, and behavioral patterns.
Creational Patterns
Creational patterns are used to create objects. They provide a way to decouple the creation of objects from their use. This makes it easier to create and manage objects, and it makes the code more reusable.
Structural Patterns
Structural patterns are used to compose objects into larger structures. They provide a way to assemble objects into complex, easy-to-understand, and maintained structures.
Behavioral Patterns
Behavioral patterns are used to define how objects interact with each other. They provide a way to coordinate the behavior of objects in a way that is easy to understand and maintain.
Python Code Samples
Here are a few examples of how design patterns can be used in Python:
- The Singleton pattern can be used to ensure that there is only one instance of a class. This can be useful for classes representing resources, such as database connections or file handles.
class Singleton:
_instance = None
@classmethod
def getInstance(cls):
if not cls._instance:
cls._instance = cls()
return cls._instance
- The Factory pattern can create objects without specifying their concrete type. This can be useful for creating objects that are configured at runtime.
class Factory:
def create(self, type):
if type == "A":
return A()
elif type == "B":
return B()
else:
raise ValueError("Invalid type")
- The Strategy pattern can be used to encapsulate a family of algorithms. This can be useful for making it easy to switch between different algorithms at runtime.
class Strategy:
def __init__(self, algorithm):
self.algorithm = algorithm
def execute(self):
return self.algorithm.execute()
Conclusion
Design patterns are a valuable tool for software developers. They can help you write better, more maintainable code by providing a shared vocabulary for discussing design problems and solutions and reusable solutions to common issues.