Diving into Python Data Types
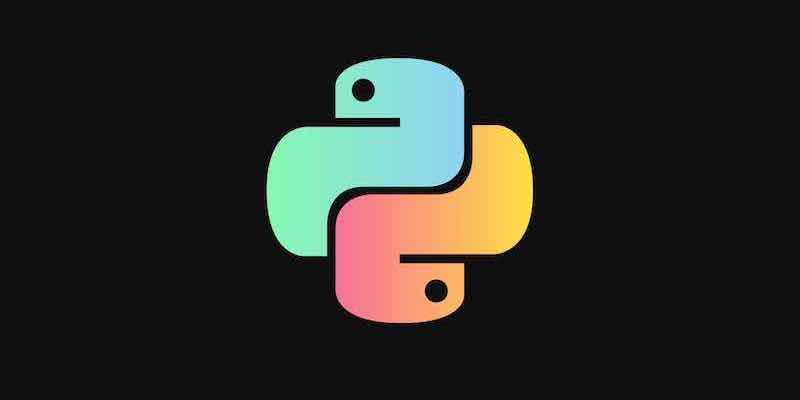
Understanding data types is fundamental to manipulating and storing data efficiently in programming. Python, a powerful and versatile language, offers a variety of built-in data types to facilitate various operations.
Python’s data types are the classifications of data items. They represent the value of what operations can be performed on a particular data. Python has several built-in data types, including numeric, sequence, mapping, set, and boolean types. Let’s delve deeper into each of these categories.
1. Numeric Types
Python supports three different numerical types:
1.1 Integers
Integers are whole numbers without a decimal point.
x = 5
y = -3
print(type(x)) # Output: <class 'int'>
1.2 Floats
Floats represent real numbers and are written with a decimal point.
x = 4.56
y = -7.89
print(type(x)) # Output: <class 'float'>
1.3 Complex Numbers
Complex numbers are written with a “j” as the imaginary part.
x = 3+5j
print(type(x)) # Output: <class 'complex'>
2. Sequence Types
Sequence types include strings, lists, and tuples, which can store a collection of items.
2.1 Strings
Strings are characters enclosed within single, double, or triple quotes.
str1 = "Hello, World!"
str2 = 'Python Programming'
print(type(str1)) # Output: <class 'str'>
2.2 Lists
Lists are ordered supplies of things (of any data type) enclosed within square brackets.
my_list = [1, 2, "Python", 4.5]
print(type(my_list)) # Output: <class 'list'>
2.3 Tuples
Tuples are ordered, immutable collections of objects enclosed within parentheses.
my_tuple = (1, 2, "Python", 4.5)
print(type(my_tuple)) # Output: <class 'tuple'>
3. Mapping Type
3.1 Dictionary
Dictionaries are unordered collections of data in a key: value pair form.
my_dict = {"name": "Alice", "age": 25}
print(type(my_dict)) # Output: <class 'dict'>
4. Set Types
Sets are unordered collections of unique items.
my_set = {1, 2, 3, 4, 5}
print(type(my_set)) # Output: <class 'set'>
5. Boolean Type
The boolean type can hold two values: True or False.
x = True
y = False
print(type(x)) # Output: <class 'bool'>
Judgment
Understanding Python’s data types is a critical step in mastering the language. The language offers a diverse range of data types to facilitate various operations and manipulations of data in your programs. This article equipped you with knowledge and code samples concerning Python data types, paving the way for you to build robust and efficient Python applications. Happy programming!