Elevating Web Development with Django: A Guide to Python’s Robust Framework
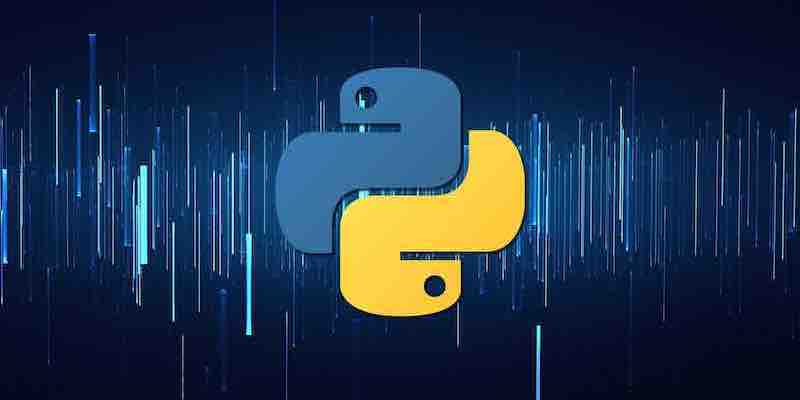
In web development, Django stands tall as one of the most popular and powerful frameworks available. Built with Python, it encourages rapid growth and clean, pragmatic design. We dive deep into Django, offering a detailed exploration complemented by code samples to help you get started with this versatile library.
Introduction
Django, affectionately known as the framework for “perfectionists with deadlines,” is revered for its simplicity, flexibility, and reliability. It follows the “Don’t Repeat Yourself” (DRY) principle, promoting code reusability and maintaining high organization and efficiency. Here, we unveil Django’s various features and functionalities, illustrating how to harness its power for your web development projects.
Setting Up Django
Before creating a Django project, install Django in your Python environment. You can install it via pip using the following command:
pip install django
Next, create a new Django project using the power:
django-admin startproject myproject
Creating a Simple Web Page
We will create a new application within our project to create a simple web page with Django. Navigate to your project’s directory (my project) and execute the following command:
python manage.py startapp myapp
Now, let’s make a view in myapp/views.py
:
from django.shortcuts import render
def home(request):
return render(request, 'home.html')
Next, create a URL pattern to point to this view in myapp/urls.py
:
from django.urls import path
from . import views
urlpatterns = [
path('', views.home, name='home'),
]
Now, create a home.html
template in the myapp/templates
directory and add some HTML content:
<!DOCTYPE html>
<html>
<head>
<title>My Django App</title>
</head>
<body>
<h1>Welcome to My Django App</h1>
</body>
</html>
Don’t forget to link the app URLs to the project URLs in myproject/urls.py
:
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('myapp.urls')), # Linking app URLs
]
Now, run the server using the command:
python manage.py runserver
Visit http://127.0.0.1:8000/
in your browser, and you should see your web page.
Building Models with Django
Django allows you to define models – Python classes that map to database tables. Here’s a simple example of a model in myapp/models.py
:
from django.db import models
class BlogPost(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
published_date = models.DateTimeField('date published')
Don’t forget to register your model in myapp/admin.py
:
from django.contrib import admin
from .models import BlogPost
admin.site.register(BlogPost)
Run migrations to create the database schema:
python manage.py makemigrations
python manage.py migrate
Summary
We’ve only scratched the surface of what Django has to offer. Its comprehensive documentation, vibrant community, and many built-in features make it a powerful tool for web development, from simple applications to complex websites. Dive in and explore Django’s capabilities; you’ll find it indispensable in your web development toolkit.