Integrating Sentry Into Python Web Applications
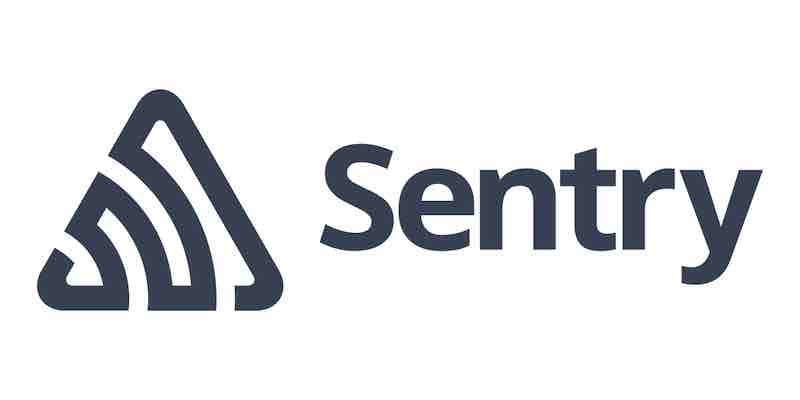
Integrating Sentry into Python web applications is straightforward. Here’s a guide on how to use Sentry with Python web applications, with a focus on Flask and Django, two popular web frameworks:
1. Installation:
First, you’ll need to install the Sentry SDK for Python:
pip install sentry-sdk
2. Integration with Flask:
If you’re using Flask, here’s how you can integrate Sentry:
from flask import Flask
import sentry_sdk
from sentry_sdk.integrations.flask import FlaskIntegration
sentry_sdk.init(
dsn="YOUR_SENTRY_DSN", # Replace with your Sentry DSN
integrations=[FlaskIntegration()]
)
app = Flask(__name__)
@app.route('/')
def hello():
return "Hello, World!"
if __name__ == '__main__':
app.run()
3. Integration with Django:
For Django applications, the integration process is slightly different:
First, install the Django integration:
pip install 'sentry-sdk[django]'
Then, in your settings.py
:
import sentry_sdk
from sentry_sdk.integrations.django import DjangoIntegration
sentry_sdk.init(
dsn="YOUR_SENTRY_DSN", # Replace with your Sentry DSN
integrations=[DjangoIntegration()],
# If you wish to capture only certain types of exceptions (optional):
ignore_errors=[django.db.utils.OperationalError],
)
4. Capturing Exceptions:
With the above configurations, Sentry will automatically capture and report exceptions in your Flask or Django application. However, if you want to capture exceptions or messages manually, you can do so:
from sentry_sdk import capture_exception
try:
a = 1 / 0
except ZeroDivisionError as e:
capture_exception(e)
5. Adding Context:
To enhance error reports, you can add user context or custom tags:
from sentry_sdk import configure_scope
with configure_scope() as scope:
scope.user = {"email": "user@example.com"}
scope.set_tag("my_custom_tag", "tag_value")
6. Testing the Integration:
To ensure that Sentry is correctly set up, you can trigger a test exception:
from sentry_sdk import last_event_id
from sentry_sdk import capture_message
@app.route('/debug-sentry')
def trigger_error():
capture_message("Sentry debug information: This is a test.")
return 'Event ID was: %s' % last_event_id()
Visiting the /debug-sentry
route will send a test message to Sentry.
Sentry provides a robust solution for error tracking in Python web applications. With just a few lines of code, developers can gain valuable insights into application errors, allowing for faster debugging and improved application reliability. Sentry can be helpful to your development toolkit, whether you’re using Flask, Django, or any other Python framework.