invalid literal for int() with base 10
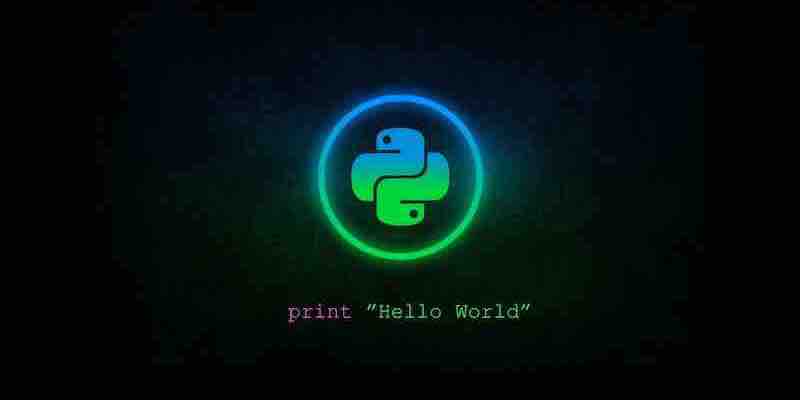
The error message invalid literal for int() with base 10: 'g'
is a standard Python error. It occurs when you try to convert a string that doesn’t represent a valid integer into an integer using the int()
function.
In this case, the string 'g'
is being passed to the int()
function, which is causing the error because 'g'
cannot be converted to an integer.
Here’s a simple example that would produce this error:
value = 'g'
integer_value = int(value)
To fix this error, ensure that the string you’re trying to convert represents a valid integer. You can handle such errors using a try-except block:
value = 'g'
try:
integer_value = int(value)
except ValueError:
print(f"'{value}' cannot be converted to an integer.")
If an invalid string is passed, the program will handle it gracefully and print a message instead of raising an exception.