Pandas AI: The Generative AI Python Library
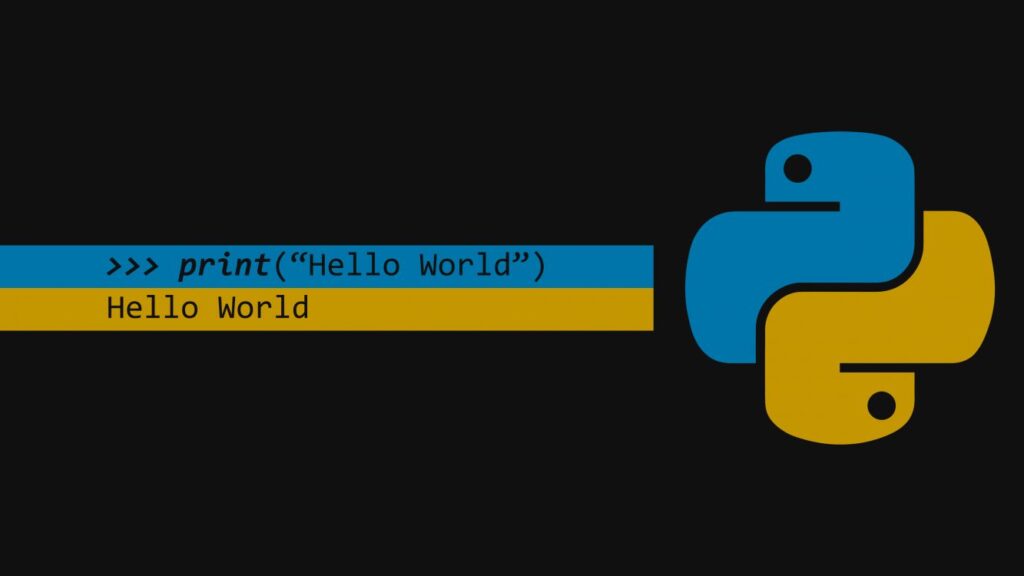
Pandas AI is a Python library that uses generative AI to create synthetic data. It is a powerful tool for data scientists and analysts who must create realistic data for testing and training machine learning models.
In machine learning, it is often necessary to create synthetic data. This is because real-world data can be challenging to obtain, expensive, and time-consuming to collect. Synthetic data can be used to train machine learning models, test the performance of machine learning models, and generate realistic data for visualization.
There are many different ways to create synthetic data. One common practice is to use a random number generator. However, arbitrary data can be unrealistic, leading to machine learning models performing poorly on real-world data.
Another way to create synthetic data is to use a generative AI model. Generative AI models are trained on real-world data and can learn to generate realistic data similar to real-world data.
What is Pandas AI?
Pandas AI is a Python library that uses generative AI to create synthetic data. Pandas AI is a powerful tool for data scientists and analysts who must create realistic data for testing and training machine learning models.
Pandas AI is built on top of the Pandas library, a powerful data manipulation and analysis library. Pandas AI makes it easy to create synthetic data compatible with Pandas DataFrames.
How to Use Pandas AI
To use Pandas AI, import the library into your Python code. You can then use the generate_data()
function to create synthetic data.
The generate_data()
the function takes a few arguments. You can specify the type of data to generate, the size of the data set, and the random seed.
The following code shows how to use the generate_data()
function to create a synthetic data set of 1000 rows and five columns:
import pandas_ai
data = pandas_ai.generate_data(
type="iris",
size=1000,
random_seed=42,
)
This code will create an artificial data set of 1000 rows and five columns. The data set will be a random sample of the Iris dataset, a well-known data set for machine learning.
Benefits of Using Pandas AI
There are some benefits to using Pandas AI. These benefits include:
- Realistic data: Pandas AI uses generative AI to create actual data. This means that the data is similar to real-world data, which can lead to machine learning models that perform better on real-world data.
- Easy to use: Pandas AI is easy to use. The
generate_data()
function takes a few arguments, and how to use it is easy to understand. - Compatible with Pandas: Pandas AI is compatible with Pandas DataFrames. This means that you can use Pandas AI to create synthetic data that can be used with Pandas for data manipulation and analysis.
Pandas AI is a powerful tool for data scientists and analysts who must create realistic data for testing and training machine learning models. Pandas AI is easy to use and compatible with Pandas DataFrames. Pandas AI is a good option if you need to create actual data.
Code Samples
Here are some code samples that show how to use Pandas AI:
import pandas_ai
# Create a synthetic data set of 1000 rows and 5 columns
data = pandas_ai.generate_data(
type="iris",
size=1000,
random_seed=42,
)
# Print the first few rows of the data set
print(data.head())
# Plot the data set
data.plot()
These code samples will create a synthetic data set of 1000 rows and five columns, print the first few rows, and plot the data set.