ULID in Python: A Basic Guide
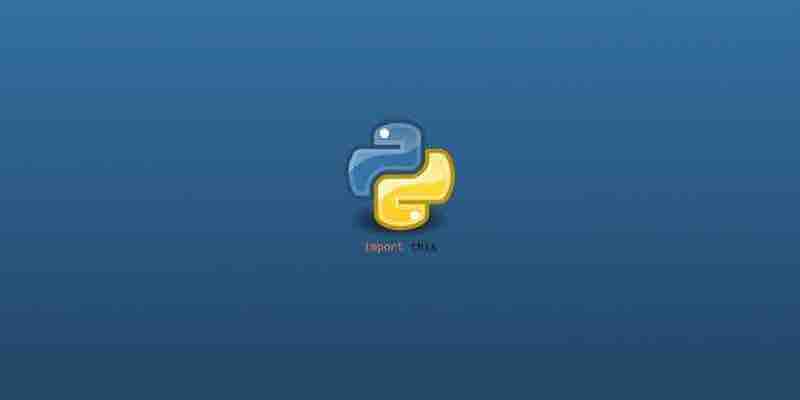
Universally unique lexicographically sortable identifiers, commonly known as ULIDs, offer a compelling alternative to traditional UUIDs. The Python package python-ulid
provides a straightforward way to work with ULIDs, ensuring compatibility, readability, and efficiency.
What is a ULID?
A ULID is a universally unique lexicographically sortable identifier. It has several distinguishing features:
- 128-bit compatibility with UUID.
- Can generate 1.21e+24 unique ULIDs per millisecond.
- Lexicographically sortable, making it ideal for databases where sorting based on creation time is essential.
- Encoded as a 26 character string, shorter than the 36 character UUID.
- Uses Crockford’s base32 for better efficiency and readability.
- Case insensitive and contains no special characters, making it URL safe.
The structure of a ULID is divided into a timestamp (48 bits) and randomness (80 bits), ensuring both uniqueness and sortability.
Getting Started with python-ulid
Installation:
To start using ULID in Python, install the package using pip:
$ pip install python-ulid
Basic Usage:
- Generate a ULID:
from ulid import ULID
new_ulid = ULID()
print(new_ulid) # Outputs: ULID(01E75HZVW36EAZKMF1W7XNMSB4)
- Generate ULID from Timestamp or Datetime:
import time, datetime
ulid_from_time = ULID.from_timestamp(time.time())
ulid_from_datetime = ULID.from_datetime(datetime.datetime.now())
- Encoding and Accessing ULID:
ulid = ULID()
print(str(ulid)) # Outputs: '01BTGNYV6HRNK8K8VKZASZCFPE'
print(ulid.hex) # Outputs: '015ea15f6cd1c56689a373fab3f63ece'
print(ulid.timestamp) # Outputs: 1505945939.153
print(ulid.datetime) # Outputs: datetime object
- Convert ULID to UUID:
uuid_version = ulid.to_uuid()
Command Line Interface
The python-ulid
The package also comes with a CLI, allowing you to generate, inspect, and convert ULIDs directly from the command line. Here are some examples:
$ ulid build # Generates a new ULID
$ ulid show 01HASFKBN8SKZTSVVS03K5AMMS # Inspects a given ULID
Conclusion
ULIDs offer a modern approach to generating unique identifiers, combining the best UUIDs with the added advantage of lexicographical sorting. The python-ulid
package makes integrating ULIDs into your Python projects easy, whether you’re building web applications, databases, or other systems that require unique, sortable IDs.
You can refer to the official documentation for more details and advanced usage,