Understanding Indexes in MySQL: Boosting Query Performance
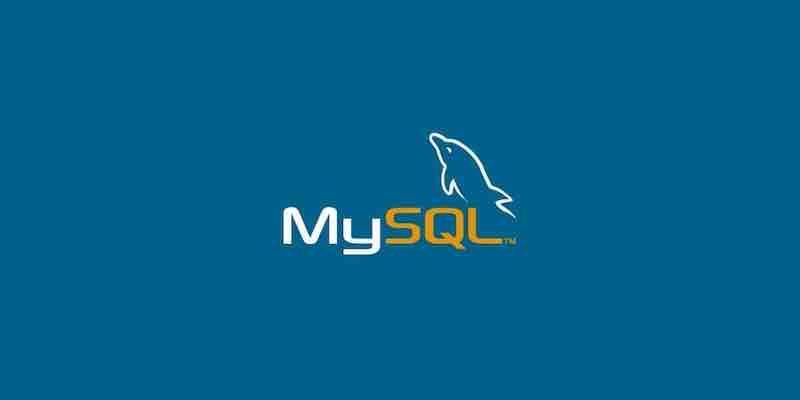
In the realm of databases, especially in MySQL, indexes play a pivotal role in enhancing the performance of search queries. Much like the index of a book that helps you quickly locate specific content, a database index allows the database system to fetch the desired data without scanning the entire table. In this article, we’ll delve deep into the concept of indexes in MySQL and illustrate their significance with query samples.
What is an Index?
An index is a data structure that improves the speed of data retrieval operations on a database table. By creating an index on one or more table columns, you can optimize the speed at which queries are processed.
Types of Indexes in MySQL
- Primary Index: This is a unique index that does not allow any duplicate values. Each table can have only one primary index. It’s typically created on the primary key.
CREATE TABLE employees (
emp_id INT PRIMARY KEY,
emp_name VARCHAR(50),
emp_age INT
);
- Unique Index: This ensures that the values in the indexed column are unique across the table.
CREATE UNIQUE INDEX idx_emp_name
ON employees (emp_name);
- Full-text Index: Used for full-text searches in text-based columns like CHAR, VARCHAR, or TEXT.
CREATE FULLTEXT INDEX idx_emp_description
ON employees (emp_description);
- Composite Index: An index that includes more than one column.
CREATE INDEX idx_emp_name_age
ON employees (emp_name, emp_age);
Benefits of Using Indexes
- Speed: The primary advantage is rapid data retrieval.
- Efficiency: Reduces the amount of data the database needs to examine.
- Performance: Enhances the performance of the
ORDER BY
clause.
When to Use Indexes
While indexes are beneficial, they aren’t always necessary. Here’s when you should consider using them:
- On columns that are frequently used in the
WHERE
clause. - On columns used in
JOIN
operations. - On columns with a wide range of unique values.
Potential Drawbacks
- Space: Indexes consume additional disk space.
- Insert/Update Overhead: Every time you modify (insert/update/delete) data in the indexed table, the index needs to be updated, which can slow down write operations.
Query Samples
- Creating an Index:
CREATE INDEX idx_emp_age
ON employees (emp_age);
- Dropping an Index:
DROP INDEX idx_emp_age ON employees;
- Viewing Indexes on a Table:
SHOW INDEXES FROM employees;
Indexes are a powerful tool in MySQL, balancing query performance and write overhead. Understanding when and how to use them can significantly optimize your database operations, ensuring efficient and rapid data retrieval. However, it’s crucial to evaluate the necessity of an index based on the specific use case and the nature of the database operations to avoid unnecessary overheads.
Creating a unique index in a database ensures that the combination of values in the indexed columns is unique across the whole table. This means that you cannot insert or update data into the table, which would result in duplicate values in the indexed columns.
Here’s how to create a unique index:
Using SQL Standard Syntax:
CREATE UNIQUE INDEX index_name
ON table_name (column1, column2, ...);
index_name
: The name you wish to give the unique index.table_name
: The name of the table on which you create the unique index.column1, column2, ...
: The columns that you want to include in the unique index.
Example:
Suppose you have a table named users
and you want to ensure that the combination of email
and username
It is unique across the table. Here’s how you’d create a unique index on those columns:
CREATE UNIQUE INDEX idx_email_username
ON users (email, username);
Using the ALTER TABLE
Syntax:
You can also create a unique index using the ALTER TABLE
Statement:
ALTER TABLE table_name
ADD UNIQUE (column1, column2, ...);
Example:
Using the same users
Table example:
ALTER TABLE users
ADD UNIQUE (email, username);
Note:
If there are already rows in the table that violate the unique constraint (i.e., they have duplicate values in the columns you’re trying to index), you’ll get an error when you try to create the unique index. You’ll need to resolve these duplicates before completing the index.