Unleashing the Power of DBSCAN: A Guide to Implementing and Understanding Clustering in Python
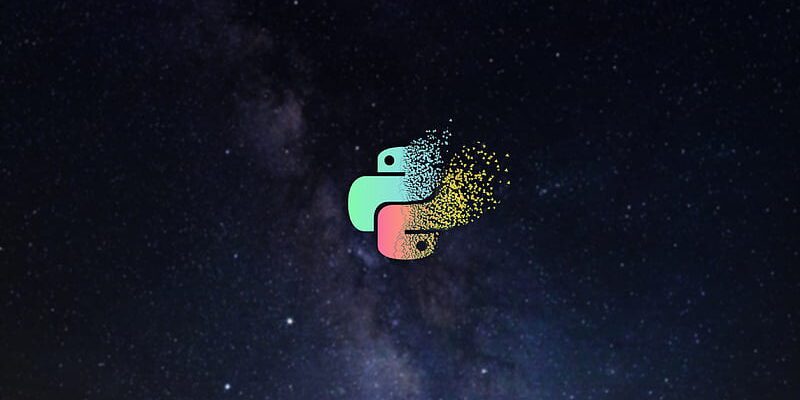
Clustering is an essential technique in data analysis and machine learning that helps to identify patterns and relationships between data points. DBSCAN (Density-Based Spatial Clustering of Applications with Noise) is one such clustering algorithm widely used in many applications due to its ability to identify clusters of different shapes and sizes.
What is DBSCAN?
DBSCAN is a density-based clustering algorithm that groups data points based on their proximity. The algorithm defines a minimum number of points (minPts) and a radius (eps) around each point. Points within this radius are considered to be part of the same cluster, while points that are farther away are considered to be outliers or noise.
DBSCAN is particularly useful when dealing with data sets with irregularly shaped clusters or clusters that are close together. It can also handle data sets with varying densities, where the density of the points in one cluster may be much higher than in another.
How does DBSCAN work?
The DBSCAN algorithm works in the following way:
Select an unvisited data point randomly and retrieve all its neighboring points within a radius eps.
If the number of neighboring points is greater than or equal to the minimum number of points minPts, a new cluster is formed, and all the neighboring points are added.
Repeat this process for all of the unvisited points until all of the points have been visited.
If a point has fewer than minPts neighboring points but is within the eps radius of another point that belongs to a cluster, it is added to that cluster.
Any points that are not visited or do not belong to any cluster are considered outliers or noise.
Implementation of DBSCAN in Python:
Python provides various libraries for implementing the DBSCAN algorithm. One such library is scikit-learn, which implements DBSCAN in its cluster module. Let’s look at how to implement DBSCAN in Python using scikit-learn:
In this example, we first generate sample data using the make_blobs function from scikit-learn ‘s datasets module. We then create an instance of the DBSCAN algorithm, specifying the values for eps and minPts. Finally, we fit the algorithm to our data and print the resulting cluster labels.
Conclusion:
In conclusion, DBSCAN is a robust clustering algorithm that can identify clusters of different shapes and sizes in data sets with varying densities. Its implementation in Python is made easy with libraries such as scikit-learn, which provide ready-made algorithm implementations. By understanding how DBSCAN works and how to implement it in Python, data analysts and machine learning practitioners can unlock new insights and understand patterns in their data.