Implementing Real-time Location Updates on Google Maps in React Native Apps
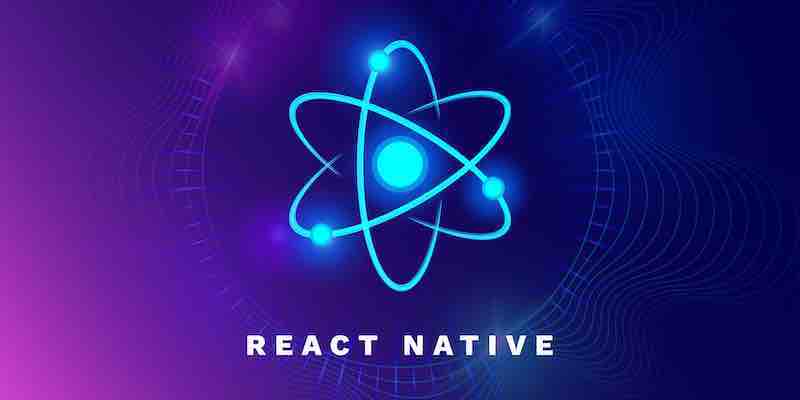
Google Maps is a powerful tool that can be used to display maps, get directions, and find businesses. In React Native, you can use the Google Maps SDK to add Google Maps to your apps.
One of the most popular features of Google Maps is the ability to track the user’s location in real-time. This can be used to create apps like ride-hailing apps, delivery apps, and fitness-tracking apps.
Prerequisites
Before you can start implementing real-time location updates, you will need to have the following:
- A Google Maps API key. You can get a free API key from the Google Maps Platform website: https://developers.google.com/maps/get-started.
- A React Native development environment. You can use any React Native IDE
Installing the Google Maps SDK
Once you have your API key, you must install the Google Maps SDK. You can do this by running the following command in your terminal:
Code snippet
npm install react-native-maps
Importing the Google Maps SDK
Once you have installed the Google Maps SDK, import it into your React Native project. You can do this by adding the following line to your package.json
file:
Code snippet
"dependencies": {
"react-native-maps": "^0.59.0"
}
Then, you need to import the SDK into your React Native code. You can do this by adding the following line to your App.js
file:
Code snippet
import React, { Component } from 'react';
import { AppRegistry, StyleSheet, Text, View, MapView } from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<MapView
style={styles.map}
initialRegion={
{
latitude: 37.774929,
longitude: -122.419418,
zoom: 13,
}
}
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
map: {
width: '100%',
height: '100%',
},
});
AppRegistry.registerComponent('App', () => App);
This code will create a basic map of the world.
Adding a Location Listener
You must add a location listener to the map to track the user’s location in real-time. The following code will add a location listener to the map:
Code snippet
import React, { Component } from 'react';
import { AppRegistry, StyleSheet, Text, View, MapView } from 'react-native';
const App = () => {
const [currentLocation, setCurrentLocation] = React.useState(null);
const onLocationChanged = (location) => {
setCurrentLocation(location);
};
return (
<View style={styles.container}>
<MapView
style={styles.map}
initialRegion={
{
latitude: 37.774929,
longitude: -122.419418,
zoom: 13,
}
}
onLocationChanged={onLocationChanged}
/>
{currentLocation && (
<Text>
Current location: {currentLocation.latitude}, {currentLocation.longitude}
</Text>
)}
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
map: {
width: '100%',
height: '100%',
},
});
AppRegistry.registerComponent('App', () => App);